Packing & unpacking are two processes that we can perform on lists in Python. We have seen the packing process in our previous article Concatenate Lists in Python, where we used packing to concatenate multiple list elements into a single list.
In this article, we will learn in depth about How to Unpack a List in Python, unpacking process on lists in Python.
Packing & Unpacking in Python
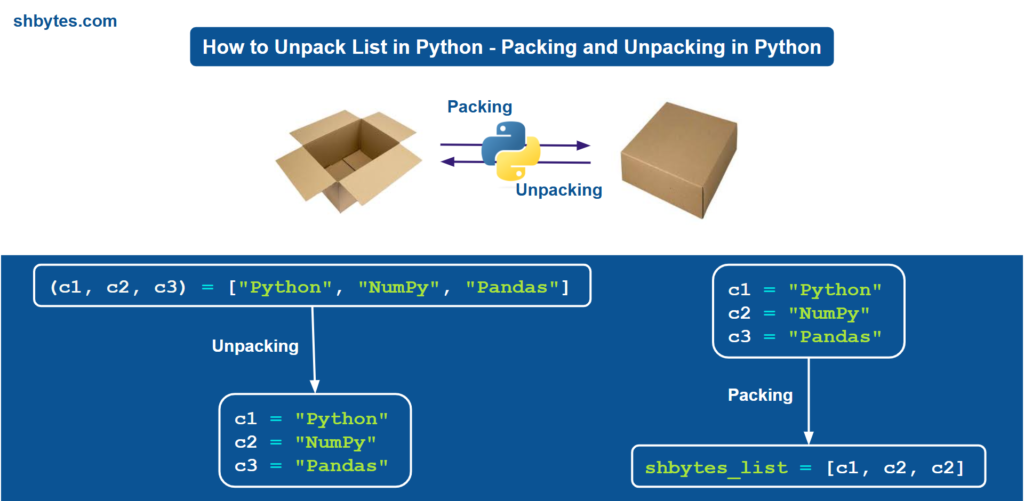
- Packing is a process of grouping multiple elements into a single list (packing)
- Unpacking is a process of extracting elements from a list (unpacking)
Python provides two operators – astrisk (*)
and double astrisk (**)
that we can use for packing and unpacking of collection elements.
astrisk (*)
is used to pack and unpack of lists & tuples.double astrisk (**)
is used to pack and unpack of dictionaries in Python.
Packing & unpacking in Python is very useful to:
- Convert list elements into separate variables. For example –
list = [10, 20]
=>a = 10, b =20
; wherea
andb
are separate variables with list element values. - Pass list elements as arguments to a function. For example
shbytes_func(*[10, 20])
;shbytes_func
is taking list elements as argument.
Let’s see different example to understand lists packing & unpacking in Python:
- Unpack List Elements Without
asterisk (*)
- Error – Unpack List without
astrisk (*)
- Unpack List – using
asterisk (*)
- Unpack List with Function Arguments
asterisk (*)
Program – Unpack List Elements Without Unpacking of list elements can be done without an astrisk (*)
. This process works only when number of elements in the list are equal to number of variables passed to extract values.
# unpack list elements
print("unpack list elements")
courses = ["NumPy", "Pandas", "Python"]
(c1, c2, c3) = courses # Unpack list elements into variable values
print("course 1 - ", c1) # c1 will be assigned the first element value from the course list
print("course 2 - ", c2) # c2 will be assigned the second element value from the course list
print("course 3 - ", c3) # c3 will be assigned the third element value from the course list
In this program, we have defined a list referenced by a variable name courses
. This list has 3 elements. During the unpacking process with (c1, c2, c3) = courses
, we are passing three variables in parentheses and assigning them to the list. Each element of the list (in order) will be assigned to each variable in the same order.
Output – Example Program – Unpack List Elements Without asterisk (*)
unpack list elements
course 1 - NumPy # c1 value - first element from unpacked list
course 2 - Pandas # c2 value - second element from unpacked list
course 3 - Python # c3 value - third element from unpacked list
From the output, the variable c1
is assigned the value NumPy
, c2
receives Pandas
, and c3
is assigned Python
. Each variable is assigned a value in the same order as the elements in the list, illustrating the order of unpacking list elements in Python.
astrisk (*)
Error Handling – Unpack List without In the previous section, we learned that unpacking list elements without astrisk (*)
can only succeed when the number of elements in the list matches the number of variables used for extraction. If the number of elements in the list does not match the number of variables, and an astrisk (*)
is not used, it will result in an error.
Program – Error Handling – Unpack List with Extra Elements
Without using an astrisk (*)
– We will get an error when the number of elements in the list are more than number of variables used for extraction. In the program below, we demonstrate an error that occurs when unpacking a list with extra elements, without using an asterisk (*).
# Error - unpacking list with extra elements
print("Error - unpacking list with extra elements")
courses = ["Python", "NumPy", "Pandas", "Java"] # List has 4 elements
try:
(a1, a2, a3) = courses # unpacking - 3 variables passed, list has 4 elements
except ValueError as err:
print("error", err)
In this program, we have defined a list courses
which has 4 elements. (a1, a2, a3) = courses
=> we attempt unpacking of the list into only 3 variables (a1, a2, a3)
, leading to a ValueError
. This gives an error because the number of elements in the list are not equal to number of variables used for extraction and asterisk (*) was also not used.
Output – Example Program – Error – Unpacking List with Extra Elements
Error - unpacking list with extra elements
error too many values to unpack (expected 3)
From the output, we got a ValueError - too many values to unpack (expected 3)
, because without using an astrisk (*)
, list has 4 elements and we passed only 3 variables.
Program – Error Handling – Unpack List with Fewer Elements
Without using an astrisk (*)
– We will get an error when the number of elements in the list are less than number of variables used for extraction. In the program below, we demonstrate an error that occurs when unpacking a list with fewer elements, without using an asterisk (*).
# Error - unpacking list with fewer elements
print("Error - unpacking list with fewer elements")
courses = ["Python", "NumPy"] # List has 2 elements
try:
(a1, a2, a3) = courses # unpacking - 3 variables passed, list has 2 elements
except ValueError as err:
print("error", err)
In this program, we have defined a list courses
which has only 2 elements. (a1, a2, a3) = courses
=> we attempt unpacking of the list into 3 variables (a1, a2, a3)
, leading to a ValueError
. This gives an error because the number of elements in the list are not equal to number of variables used for extraction and asterisk (*) was also not used.
Output – Example Program – Error – Unpack List with Fewer Elements
Error - unpacking list with fewer elements
error not enough values to unpack (expected 3, got 2)
From the output, we got a ValueError - not enough values to unpack (expected 3, got 2)
asterisk (*)
to Unpack List Elements
Using We can unpack the elements of a list using astrisk (*)
.
- Using an
astrisk (*)
, we can have more elements in the list compare to the number of variables passed. - An
astrisk (*)
will not work if there are fewer elements in the list than the number of variables passed. - The
astrisk (*)
can be used with the first variable, any variable in between, or the last variable. - We can use an
astrisk (*)
with only 1 variable and cannot use it with multiple variables. - A variable with an
astrisk (*)
will take extra elements from the given list as a new list, which are not taken by other variables. - An
astrisk (*)
always returns a list, whether unpacked from a tuple or a list.
asterisk (*)
on the First Variable
Program – Unpack List using Unpacking the list with an astrisk (*)
– We can use an astrisk (*)
with the first variable.
# unpacking list - using asterisk* first variable
print("unpacking list - using asterisk* first variable")
courses = ["NumPy", "Pandas", "Python", "Java", "Data Science", "Machine Learning"]
(*c1, c2, c3) = courses # unpacking, astrisk (*) used with first variable
print("course list c1 - ", c1) # c1 with astrisk, will take the extra elements from the list
print("course c2 - ", c2) # c2 will be assigned with second-last element of the list
print("course c3 - ", c3) # c3 will be assigned with last element of the list
The defined list courses
has 6 elements. During the unpacking process, we are passing 3 variables (*c1, c2, c3)
. The variable c1
uses an asterisk (*)
. Since the list has more elements than the variables passed, the variables c2
and c3
will take the last two elements (in order) from the list, while the remaining elements are assigned to the variable c1
as a list.
Output – Example Program – Unpack List using asterisk (*) on the First Variable
unpacking list - using asterisk* first variable
course list c1 - ['NumPy', 'Pandas', 'Python', 'Java'] # all elements (except last two elements) from the list
course c2 - Data Science # second-last element of the list
course c3 - Machine Learning # last elements of the list
In the program output, variable c2
and c3
are assigned last two elements (in order) from the list and c1
is the list with remaining elements.
asterisk (*)
in an In-Between Variable
Program – Unpack List using Unpacking a list with an astrisk (*)
– We can use an astrisk (*)
with any of the in-between variables.
# unpacking list - using asterisk* in between
print("unpacking list - using asterisk* in between")
courses = ["NumPy", "Pandas", "Python", "Java", "Data Science", "Machine Learning"]
(c1, *c2, c3) = courses # unpacking, astrisk (*) used with in-between variable
print("course c1 - ", c1) # c1 will be assigned with first element of the list
print("course list c2 - ", c2) # c2 will be assigned with all in-between elements of the list
print("course c3 - ", c3) # c3 will be assigned with last element of the list
The defined list courses
has 6 elements. (c1, *c2, c3) = courses
=> During the unpacking process, we are passing 3 variables (c1, *c2, c3)
. The variable c2
uses an asterisk (*)
. Since the list has more elements than the variables passed, the variable c1
is assigned the first element of the list, and c3
is assigned the last element. The remaining in-between elements are assigned to the variable c2
as a list.
Output – Example Program – Unpack List using asterisk (*) in an In-Between Variable
unpacking list - using asterisk* in between
course c1 - NumPy # first elements of the list
course list c2 - ['Pandas', 'Python', 'Java', 'Data Science'] # all in-between elements of the list
course c3 - Machine Learning # last elements of the list
In the program output, variable c1
is assigned the first element of the list and c3
is assigned last element of the list and c2
is the list with remaining (in-between) elements.
asterisk (*)
on the Last Variable
Program – Unpack List using Unpacking a list with an astrisk (*)
– We can use an astrisk (*)
with the last variable.
# unpacking list - using asterisk* last variable
print("unpacking list - using asterisk* at last")
courses = ["NumPy", "Pandas", "Python", "Java", "Data Science", "Machine Learning"]
(c1, c2, *c3) = courses # unpacking, astrisk (*) used with last variable
print("course c1 - ", c1) # c1 will be assigned with first element of the list
print("course c2 - ", c2) # c2 will be assigned with second element of the list
print("course list c3 - ", c3) # c3 will be assigned with all extra elements of the list
Again, defined list ‘courses’ has 6 elements. During the unpacking process, we are passing 3 variables (‘c1’, ‘c2’, ‘*c3’). Variable ‘*c3’ is with astrisk (*). List has more number of elements compared to number of variables passed. Variable ‘c1’ will be assigned first element of list and ‘c2’ will be assigned second element of list and remaining last elements will be assigned to variable ‘c3’ as a list.
The defined list courses
has 6 elements. (c1, c2, *c3) = courses
=> During the unpacking process, we are passing 3 variables (c1, c2, *c3)
. The variable c3
uses an asterisk (*)
. Since the list has more elements than the variables passed, the variable c1
is assigned the first element of the list, c2
is assigned the second element, and the remaining elements are assigned to the variable c3
as a list.
Output – Example Program – Unpack List using asterisk (*) on the Last Variable
unpacking list - using asterisk* at last
course c1 - NumPy # first elements of the list
course c2 - Pandas # second elements of the list
course list c3 - ['Python', 'Java', 'Data Science', 'Machine Learning'] # all extra elements of the list
In the program output, variable c1
is assigned the first element of the list and c2
is assigned the second element of the list and c3
is the list with remaining (last) elements.
asterisk (*)
Multiple Times
Example: Error Handling – Unpack List using We will get a SyntaxError
if we use an asterisk (*)
with multiple variables during list unpacking.
# Error - unpacking list - using asterisk* multiple times
print("Error - unpacking list - using asterisk* multiple times")
courses = ["NumPy", "Pandas", "Python", "Java", "Data Science", "Machine Learning"]
try:
(c1, *c2, *c3) = courses # unpacking, astrisk (*) used with multiple variables
except SyntaxError as err:
print("error", err)
(c1, *c2, *c3) = courses
=> During the unpacking process, we are passing 3 variables (c1, *c2, *c3)
. Variables c2
& c3
is with astrisk (*)
. Because of multiple variables with astrisk(*)
, program will not know which variable to assigned the remaining (extra) elements. That is why it will throw an error – SyntaxError: multiple starred expressions in assignment
.
Output – Example Program – Error – Unpack List using asterisk (*) Multiple Times
File "D:-unpacking-list.py", line 63
(c1, *c2, *c3) = courses
^^^^^^^^^^^^^^
SyntaxError: multiple starred expressions in assignment
Unpack List with Function Arguments
In previous sections, we have seen how unpacking process works and how we can use astrisk (*)
to unpack multiple elements of a list. Generally, unpacking process is used to pass the list elements as an argument to a function. Lets understand this with program.
Program – Unpack List with Function Arguments
In this program, we defined a function fun_print_arguments
with 4 arguments (k, l, m, n)
. We also define a list number_list
, which initially contains 5 elements.
# Unpacking list with function arguments
print("Unpacking list with function arguments\n")
def fun_print_arguments(k, l, m, n):
print("Number k - ", k)
print("Number l - ", l)
print("Number m - ", m)
print("Number n - ", n)
Three scenarios we are testing with this program:
asterisk (*)
)
Scenario 1: Passing List Directly to the Function (without If a list is passed directly to the function without using an asterisk (*)
, it will result in an error.
number_list = [30, 40, 50, 60, 70]
# Passing list directly to function will give an error
print("\nPassing list directly to function will give an error")
try:
fun_print_arguments(number_list) # passing list without astrisk (*)
except TypeError as err:
print("error", err)
In this scenario, fun_print_arguments(number_list)
=> the list is passed directly without unpacking, which results in a TypeError
because the function expects 4 arguments but receives only 1. Passing list directly will not unpack its elements and will be considered as a single argument passed. The error message will be fun_print_arguments() missing 3 required positional arguments: 'l', 'm', and 'n'
.
astrisk (*)
) Passing List having More Elements Than Function Arguments
Scenario 2: (withIf the list contains more elements than the number of function arguments is passed as a function argument with an astrisk (*)
, it will result in an error.
number_list = [30, 40, 50, 60, 70]
# Passing more elements than function arguments will give an error
print("\nPassing more elements than function arguments will give an error")
try:
fun_print_arguments(*number_list) # passing list has more number of elements
except TypeError as err:
print("error", err)
In this scenario, we pass a list with astrisk (*)
as an argument. This list has 5 elements, but the function expects only 4 arguments. Passed list will be unpacked with 5 elements but will fail to assign all elements to variables. This causes a TypeError: fun_print_arguments() takes 4 positional arguments but 5 were given
astrisk (*)
) Passing the Same Number of Elements as Function Arguments
Scenario 3: (withWhen the list contains the same number of elements as required by the function, the unpacking works correctly.
# Passing same number elements as function arguments
print("\nPassing same number elements as function arguments")
number_list = [30, 40, 50, 60]
fun_print_arguments(*number_list) # passing list has same number of elements
In this scenario, we pass a list with astrisk (*)
as an argument. The list has 4 elements, which matches the 4 arguments expected by the function. Passed list will be unpacked with 4 elements and all elements will be assigned to variables. The unpacking happens successfully, and the values are printed as expected.
Complete Program Output (including all sections)
Unpacking list with function arguments
Passing list directly to function will give an error
error fun_print_arguments() missing 3 required positional arguments: 'l', 'm', and 'n'
Passing more elements than function arguments will give an error
error fun_print_arguments() takes 4 positional arguments but 5 were given
Passing same number elements as function arguments
Number k - 30
Number l - 40
Number m - 50
Number n - 60
Summary
In this article we learned various ways to pack and unpack list elements in Python. We covered:
- Packing & Unpacking in Python
- Unpack List Elements Without
asterisk (*)
- Error Handling: Unpack List with Extra Elements
- Error Handling: Unpack List with Fewer Elements
- Using
asterisk (*)
to Unpack List Elements - Unpack List using
asterisk (*)
on the First Variable - Unpack List using
asterisk (*)
in an In-Between Variables - Unpack List using
asterisk (*)
on the Last Variable - Error Handling – Unpack List using
asterisk (*)
Multiple Times - Unpack List with Function Arguments
Code snippets and programs related to How to Unpack a List in Python, can be accessed from GitHub Repository. This GitHub repository all contains programs related to other topics in Python tutorial.
Interview Questions & Answers
Q: How would you unpack a nested list?
To unpack a nested list, we have assign variables to the inner lists, according the inner list elements.
nested_list = [11, [21, 23, 34], [34, 45, 56]]
a, (*b, c), (d, *e) = nested_list
print(a) # value of a = 11
print(b) # value of b = [21, 23]
print(c) # value of c = 34
print(d) # value of d = 34
print(e) # value of e = [45, 56]
- In this example,
a
captures the first element. (*b, c)
unpacks the second inner list andc
captures the last element of that inner list andb
(with *)captures other elements(d, *e)
unpacks the third inner list andd
captures the first element ande
(with *) captures all other elements
Q: Explain how to use packing and unpacking in function arguments?
Packing and unpacking are very useful in functions, particularly when number of arguments are not defined.
- Packing in Function Arguments – We can use the
*
operator to pack multiple arguments into a single tuple.
def func(*args):
print(args) # *args captures all positional arguments into a tuple.
func(11, 12, 34) # print from func arguments = (11, 12, 34)
- Unpacking in Function Arguments – We can unpack a list or a tuple directly into function arguments using the
*
operator.
def add(x, y, z):
return x + y + z
values = [11, 21, 34]
result = add(*values) # Unpacks list into 3 arguments
print(result) # Total sum of elements: 66
In this example, *values
unpacks the list values
into three separate arguments, which are passed to the add
function.
Q: What are some common errors associated with unpacking in Python?
- Mismatch in number of variables and list items – If the number of variables on the left side of the unpacking operation does not match the number of elements in the list or tuple, Python will raise a
ValueError
. Example –a, b = [12, 14, 42] # Raises ValueError: too many values to unpack
- Using the
*
operator incorrectly – The*
operator must be used correctly within the context of the unpacking. Using it in the wrong position or without understanding its behavior can lead to logical errors or exceptions. Example –*a, b = [12, 14, 42]
anda, *b = [12, 14, 42]
, both are valid assignments but*
operator with different variables. - Unpacking with incompatible data types – Ensure that the structure of the list or tuple matches the expected structure during unpacking. For example, trying to unpack a single-level list into nested variables will raise an error. Example –
a, (b, c) = [12, 14, 42] # Raises ValueError: not enough values to unpack
*
and double asterisk **
in Python function definitions?
Q: What is the difference between single asterisk Single asterisk *
is used to pack positional arguments into a tuple. Example: def func(*args):
allows the function to accept any number of positional arguments, which will be packed into the args
tuple.
Double asterisk **
is used to pack keyword arguments into a dictionary. Example: def func(**kwargs):
allows the function to accept any number of keyword arguments, which will be packed into the kwargs
dictionary.
def func(**kwargs):
print(kwargs)
func(a=12, b=22, c=32) # kwargs elements - {'a': 12, 'b': 22, 'c': 32}
Both *
and **
can be used together to allow a function to accept an arbitrary number of both positional and keyword arguments.
def func(*args, **kwargs):
print(args)
print(kwargs)
func(12, 22, 32, a=44, b=54) # args elements - (1, 2, 3) # kwargs elements - {'a': 4, 'b': 5}
Test Your Knowledge: Practice Quiz
Related Topics
- Count & Sort Methods in Python Lists: Tutorial with ExamplesThe count() & Sort() methods are built-in methods in Python lists, useful for counting occurrences of elements and sorting of elements in the list. Lists in Python can store duplicate elements and elements of various data types, making these methods essential for data handling. In previous article, we learned about Loops and List Comprehension in Python.…
- Loops and List Comprehension in Python: A Complete GuideLooping is a process to repeat the same task multiple times. It provides sequential access to elements in the list. Comprehension offers a shorter syntax to iterate (loop) through list elements. In previous article, we learned about How to Unpack List in Python. In this article we will learn about Python list loops and List…
- How to Unpack List in Python – Packing and Unpacking in Python (with Programs)Packing & unpacking are two processes that we can perform on lists in Python. We have seen the packing process in our previous article Concatenate Lists in Python, where we used packing to concatenate multiple list elements into a single list. In this article, we will learn in depth about How to Unpack a List…
- Concatenate Lists in Python – Complete Guide to List Concatenation MethodsConcatenation of lists in Python refers to merging of elements from two or more lists into a single list. Python provides a variety of methods for list concatenation, allowing flexibility based on the requirements of each task. For all topics related to lists, check out the Lists in Python: A Comprehensive Guide guide. In the…
- Introduction to List Slicing in Python – Methods, Syntax, and ExamplesList slicing in Python or slicing of list in Python means extracting a part (or slice) of the list. Slicing of list uses the index position of elements in the list. Slicing can be done using either of two methods: In previous articles, we learned about accessing list elements by index, using the list index method to…