Python list elements are stored based on their index positions and can be accessed by their index positions, which allows to quickly retrieve specific element from the list. Index assignment to list element in Python, is similar to index assigned in Array elements. Understanding how to access list elements by index is crucial for data manipulation in Python.
This article covers all topics related to access list elements by index in Python along with positive and negative indexing methods and how to handle errors like IndexError: list index out of range
. Read tutorial to learn more about – How to Create List in Python.
Index of an element in list
List elements in Python are stored with index positions, similar to array elements. Python lists offer two ways to index elements:
- Index from left to right (Positive Indexing) – Counts from left to right, starting at index 0.
- Index from right to left (Negative Indexing) – Counts from right to left, starting at index -1.
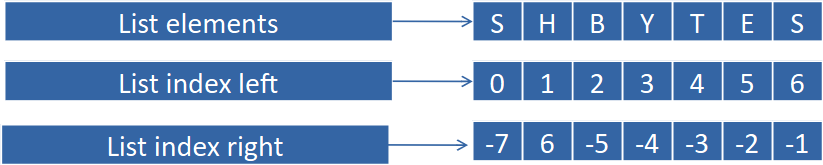
Index from left to right (Positive Indexing)
Index of an element in the list starts from left to right. Left most element i.e. first element is assigned index 0 and then index increases one-by-one in the right direction. The right most element i.e. last element of the list will have index = number of element in list -1.
This indexing from left to right also known as Positive Indexing.
As shown in the above diagram, List elements are [‘S’, ‘H’, ‘B’, ‘Y’, ‘T’, ‘E’, ‘S’]. Total number of elements in the list are 7. First element i.e. ‘S’ will have index 0 and then the index of each elements towards the right will increase by 1. Last element i.e. ‘S’ again will have index 6.
Index from right to left (Negative Indexing)
Index of an element in the list can also starts from right to left. Right most element i.e. last element is assigned index -1 and then index decreased one-by-one in the left direction. The left most element i.e. first element of the list will have index = negative of number of element in list.
This indexing from right to left also known as Negative Indexing.
As shown in the above diagram, List elements are [‘S’, ‘H’, ‘B’, ‘Y’, ‘T’, ‘E’, ‘S’]. Total number of elements in the list are 7. Last element i.e. ‘S’ will have index -1 and then index for each elements towards left will decrease by 1. First element i.e. ‘S’ again will have index -7.
Access List Elements by Index
There are multiple methods based on index position, using which we can access list elements.
- Access List Elements using Positive Indexing
- Traverse List using Positive Indexing
- Access List Elements using Negative Indexing
- Traverse List using Negative Indexing
IndexError
– List Index out of range
Access List Elements using Positive Indexing
# Access List Elements using positive indexing, start from left, start with 0
shbytes_list = ["Java", "Azure", "AWS", "Python", 14, 15, 16]
print(shbytes_list)
print("element at index 0 - ", shbytes_list[0])
print("element at index 1 - ", shbytes_list[1])
print("element at index 5 - ", shbytes_list[5])
We have defined a list containing both string and number elements. This list is referenced by a variable name shbtyes_list
and contains a total of 7 elements. Each element in the list is assigned a positive index from left to right. To access an element, we use the list variable name along with its index position. The Left most element i.e. the first element, can be accessed based on its index 0 and subsequent elements to the right follow incrementally.
Output – Example Program – Access List Elements using Positive Indexing
['Java', 'Azure', 'AWS', 'Python', 14, 15, 16]
element at index 0 - Java
element at index 1 - Azure
element at index 5 - 15
At index 0 is the left most element i.e. first element “Java” and then other index elements are printed.
Traverse List using Positive Indexing
# Traverse List using Positive Indexing, start from left, start with 0
shbytes_list = ["Java", "Azure", "AWS", "Python", 14, 15, 16]
print(shbytes_list)
for i in range(len(shbytes_list)):
print("index : ", i, " | value : ", shbytes_list[i])
In this example program, we access list elements using positive indexing. We are using the for
loop to dynamically access each index position and get the element at that index. The range
and len
built-in functions are used to iterate through the index positions, ensuring the we access all elements in the list from left to right.
Output – Example Program – Traverse List using Positive Indexing
['Java', 'Azure', 'AWS', 'Python', 14, 15, 16]
index : 0 | value : Java
index : 1 | value : Azure
index : 2 | value : AWS
index : 3 | value : Python
index : 4 | value : 14
index : 5 | value : 15
index : 6 | value : 16
In the output, all elements of the list are printed based on their index position. Elements were iterated from left to right. First element at index 0 is printed and then other elements.
Access List Elements using Negative Indexing
# Access List Elements using negative indexing, start from left, start with 0
shbytes_list = ["Java", "Azure", "AWS", "Python", 14, 15, 16]
print(shbytes_list)
print("element at index -1 - ", shbytes_list[-1])
print("element at index -2 - ", shbytes_list[-2])
print("element at index -7 - ", shbytes_list[-7])
In this example program, we have defined a list referenced by a variable name shbytes_list
and consists of 7 elements. This list elements will be assigned negative indexes starting from the right to the left. To access any element, we reference the list variable and use the corresponding negative index. The rightmost element i.e. last element can be accessed with index -1 and can access other elements towards left with decreasing index positions.
Output – Example Program – Access List Elements using Negative Indexing
['Java', 'Azure', 'AWS', 'Python', 14, 15, 16]
element at index -1 - 16
element at index -2 - 15
element at index -7 - Java
At index -1 is the right most element i.e. last element 16 and then other index elements are printed.
Traverse List using Negative Indexing
# Traverse List using Positive Indexing, start from right, start with -1
shbytes_list = ["Java", "Azure", "AWS", "Python", 14, 15, 16]
print(shbytes_list)
for i in range(-1, -(len(shbytes_list)+1), -1):
print("index : ", i, " | value : ", shbytes_list[i])
In this example program, we will access list elements using negative indexing. In this case, we are using a for
loop to dynamically get the index positions. The built-in range
and len
built-in functions are used to iterate through the list’s index positions. The range
function starts at index -1 and decreases the index by -1 with each iteration. This process continues till it reach the length of the list.
Output – Example Program – Traverse List using Negative Indexing
['Java', 'Azure', 'AWS', 'Python', 14, 15, 16]
index : -1 | value : 16
index : -2 | value : 15
index : -3 | value : 14
index : -4 | value : Python
index : -5 | value : AWS
index : -6 | value : Azure
index : -7 | value : Java
In the output, all elements of the list are printed based on their negative index positions. The elements were iterated from right to left, starting with the element at index -1 followed by other elements in reverse order.
IndexError
– List Index out of range
Positive index or negative index, both are assigned as per the number of elements present in the list. If we pass an out of range index position for the list, then we will get an error.
IndexError
with Positive Index
# Error out of range - positive index
shbytes_list = ["Java", "Azure", "AWS", "Python", 14, 15, 16]
print(shbytes_list)
print("element at index 7 - ", shbytes_list[7])
This list has total of 7 elements. Positive indexing will be from 0 to 6. Index 7 is out of this range.
Program Output
Traceback (most recent call last):
File "D:-index-element-list.py", line 36, in <module>
print("element at index 7 - ", shbytes_list[7])
~~~~~~~~~~~~^^^
IndexError: list index out of range
Index 7 is not present for the given list. So, it will raise an IndexError: list index out of range
IndexError
with Negative Index
# Error out of range - negative index
shbytes_list = ["Java", "Azure", "AWS", "Python", 14, 15, 16]
print(shbytes_list)
print("element at index -8 - ", shbytes_list[-8])
Total 7 elements are present in the given list. Negative indexing will be from -1 to -7. Index -8 is out of this range.
Program Output
Traceback (most recent call last):
File "D:-index-element-list.py", line 35, in <module>
print("element at index -8 - ", shbytes_list[-8])
~~~~~~~~~~~~^^^^
IndexError: list index out of range
Index -8 is not present for the given list. So, it gave an IndexError: list index out of range
Summary
In Python, list elements can be accessed using positive indexing (from left to right) or negative indexing (from right to left). Positive indexing starts at 0
for the first element (from left), while negative indexing starts at -1
for the first element (from right). You can access individual elements by directly specifying their index position or loop through the list using a for
loop with the help of range()
and len()
functions.
While working with list indexing, it’s crucial to avoid IndexError
by ensuring the index is within the valid range: 0 to len(list)-1
for positive indexing and -len(list) to -1
for negative indexing.
This guide explains how to use both positive and negative indexing to efficiently access and traverse Python lists.
Code snippets and programs related to Access List Elements by Index in Python, can be accessed from GitHub Repository. This GitHub repository all contains programs related to other topics in Python tutorial.
Interview Questions & Answers
Q: What happens if we access an index that is out of range in a list?
Out of range index cannot be accessed from the list. Python raises an IndexError
.
my_list = [1, 2, 3] # This array only 3 elements, index from 0 to 2
print(my_list[5]) # Raises IndexError: list index out of range
shbytes_list[2]
and shbytes_list[2:3]
?
Q: Difference between shbytes_list[2]
accesses a single element at index2
and returns that element.shbytes_list[2:3]
returns a slice containing the element at index2
. The result is a list containing that single element.
my_list = [10, 20, 30, 40]
print(my_list[2]) # Output: 30
print(my_list[2:3]) # Output: [30]
Q: What are some common errors when accessing list elements by index?
- IndexError – This error occurs when we try to access an index that is out of range.
- TypeError – This error happens when we try to access an index with a non-integer value.
- Negative Index Misuse – This happens when we use negative indices incorrectly. This in case when the list is empty or when we expect positive index behavior.
course_list = ['Python', 'PowerBI', 'NumPy']
element = course_list['one'] # TypeError: list indices must be integers or slices, not str
Test Your Knowledge: Practice Quiz
Related Topics
- Count & Sort Methods in Python Lists: Tutorial with ExamplesThe count() & Sort() methods are built-in methods in Python lists, useful for counting occurrences of elements and sorting of elements in the list. Lists in Python can store duplicate elements and elements of various data types, making these methods essential for data handling. In previous article, we learned about Loops and List Comprehension in Python.…
- Loops and List Comprehension in Python: A Complete GuideLooping is a process to repeat the same task multiple times. It provides sequential access to elements in the list. Comprehension offers a shorter syntax to iterate (loop) through list elements. In previous article, we learned about How to Unpack List in Python. In this article we will learn about Python list loops and List…
- How to Unpack List in Python – Packing and Unpacking in Python (with Programs)Packing & unpacking are two processes that we can perform on lists in Python. We have seen the packing process in our previous article Concatenate Lists in Python, where we used packing to concatenate multiple list elements into a single list. In this article, we will learn in depth about How to Unpack a List…
- Concatenate Lists in Python – Complete Guide to List Concatenation MethodsConcatenation of lists in Python refers to merging of elements from two or more lists into a single list. Python provides a variety of methods for list concatenation, allowing flexibility based on the requirements of each task. For all topics related to lists, check out the Lists in Python: A Comprehensive Guide guide. In the…
- Introduction to List Slicing in Python – Methods, Syntax, and ExamplesList slicing in Python or slicing of list in Python means extracting a part (or slice) of the list. Slicing of list uses the index position of elements in the list. Slicing can be done using either of two methods: In previous articles, we learned about accessing list elements by index, using the list index method to…