Elements in the tuple are stored based on their index positions. Index assignment to tuple elements is similar to index assigned in Array elements. We can access tuple elements by index index positions. Let’s first understand how index positions are assigned to tuple elements.
Read article, to learn more about How to Access List Elements by Index in Python.
Index of an Element in Tuple
Elements in the tuple are stored based on their index positions. Index assignment to tuple elements is similar to index assignment in array elements. Tuple elements are assigned indexes in two ways:
- Index from Left to Right (Positive Indexing)
- Index from Right to Left (Negative Indexing)
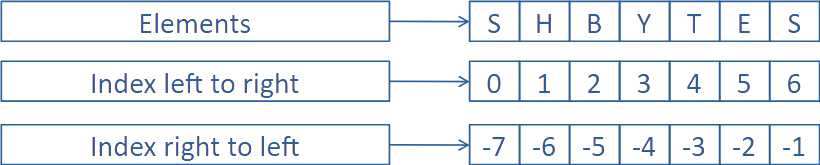
Index from Left to Right (Positive Indexing)
The index of an element in the tuple starts from left to right. The leftmost element, i.e., the first element, is assigned index 0
, and then the index increases one-by-one in the right direction. The rightmost element, i.e., the last element of the tuple, will have an index equal to the number of elements in the tuple minus 1.
This indexing from left to right is also known as Positive Indexing.
As shown in the diagram, tuple elements are ['S', 'H', 'B', 'Y', 'T', 'E', 'S']
. The total number of elements in the tuple is 7. The first element 'S'
will have index 0
, and then the index for each element towards the right will increase by 1. The last element 'S'
will have index 6
.
Index from Right to Left (Negative Indexing)
The index of an element in the tuple can also start from right to left. The rightmost element, i.e., the last element, is assigned index -1
, and then the index decreases by 1 towards the left. The leftmost element, i.e., the first element of the tuple, will have an index equal to the negative of the number of elements in the tuple.
This indexing from right to left is also known as Negative Indexing.
As shown in the diagram, tuple elements are ['S', 'H', 'B', 'Y', 'T', 'E', 'S']
. The total number of elements in the tuple is 7. The last element 'S'
will have index -1
, and then the index for each element towards the left will decrease by 1. The first element 'S'
will have index -7
.
Access Tuple Elements by Index
There are multiple methods based on index positions, using which we can access tuple elements:
- Using Positive Indexing
- Traverse Tuple using Positive Indexing
- Using Negative Indexing
- Traverse Tuple using Negative Indexing
- Error – Index Out of Range
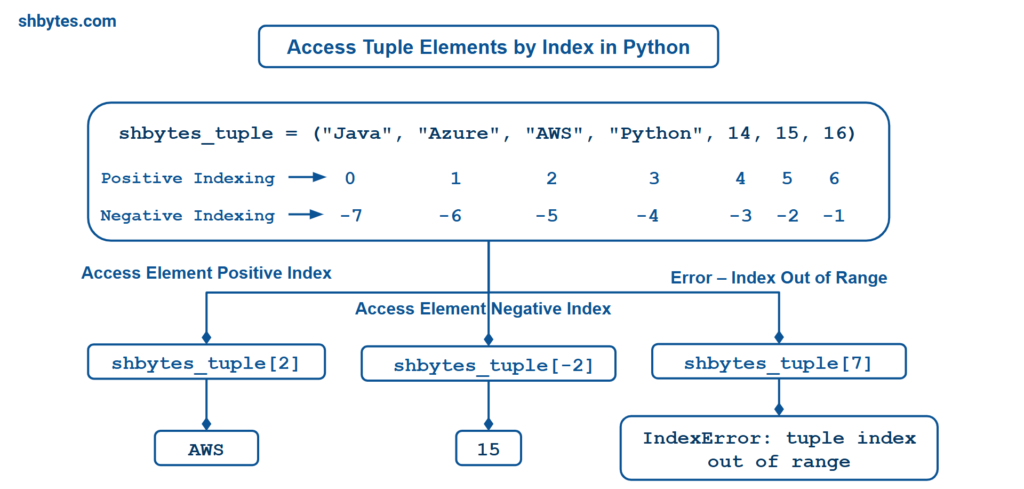
Using Positive Indexing
# Positive Indexing, start from left, start with 0
shbytes_tuple = ("Java", "Azure", "AWS", "Python", 14, 15, 16)
print(shbytes_tuple)
print("element at index 0 - ", shbytes_tuple[0])
print("element at index 1 - ", shbytes_tuple[1])
print("element at index 5 - ", shbytes_tuple[5])
We have defined a tuple of string and number elements. This tuple is referenced by the variable name shbytes_tuple
and contains 7 elements. These tuple elements will be assigned positive indexes from left to right. To access any element, we pass the tuple variable name and element index. The leftmost element, i.e., the first element, can be accessed using index 0
, and so on.
Output – Example Program – Using Positive Indexing
('Java', 'Azure', 'AWS', 'Python', 14, 15, 16)
element at index 0 - Java
element at index 1 - Azure
element at index 5 - 15
Leftmost element is at index 0
i.e. first element Java
and then subsequent index elements are printed.
Traverse Tuple using Positive Indexing
# Traverse Tuple using Positive Index, start from left at index 0
shbytes_tuple = ("Java", "Azure", "AWS", "Python", 14, 15, 16)
print(shbytes_tuple)
for i in range(len(shbytes_tuple)):
print("index : ", i, " | value : ", shbytes_tuple[i])
In this example program, we are dynamically accessing the tuple elements using the for
loop and iterating through positive index positions. We are using built-in range()
and len()
functions to generate the positive sequence numbers.
Output – Example Program – Traverse Tuple using Positive Indexing
('Java', 'Azure', 'AWS', 'Python', 14, 15, 16)
index : 0 | value : Java
index : 1 | value : Azure
index : 2 | value : AWS
index : 3 | value : Python
index : 4 | value : 14
index : 5 | value : 15
index : 6 | value : 16
From the program output, all elements of the tuple are printed based on their positive index position. Elements were iterated from left to right. First element at index 0
is printed and then subsequent elements towards right.
Using Negative Indexing
# negative index, start from right, start with -1
shbytes_tuple = ("Java", "Azure", "AWS", "Python", 14, 15, 16)
print(shbytes_tuple)
print("element at index -1 - ", shbytes_tuple[-1])
print("element at index -2 - ", shbytes_tuple[-2])
print("element at index -7 - ", shbytes_tuple[-7])
In this example program, we have defined a tuple of string and number elements referenced by the variable name shbytes_tuple
and contains 7 elements. These tuple elements will be assigned negative indexes from right to left. To access any element, we pass the tuple variable name and element’s negative index. The rightmost element, i.e., the last element, can be accessed using index -1
, and so on other elements towards left.
Output – Example Program – Using Negative Indexing
('Java', 'Azure', 'AWS', 'Python', 14, 15, 16)
element at index -1 - 16
element at index -2 - 15
element at index -7 - Java
At index -1
is the rightmost element i.e. last element 16 and then other elements towards left are printed using negative index.
Traverse Tuple using Negative Indexing
# traversing tuple using index, start from right, start with -1
shbytes_tuple = ("Java", "Azure", "AWS", "Python", 14, 15, 16)
print(shbytes_tuple)
for i in range(-1, -(len(shbytes_tuple)+1), -1):
print("index : ", i, " | value : ", shbytes_tuple[i])
In this example program, we are dynamically accessing the tuple elements using the for
loop and iterating through negative index positions. We are using built-in range()
and len()
functions to generate the negative sequence numbers. In the range()
function we are starting with -1
index and then decreasing the index by -1 till the length of the tuple.
Output – Example Program – Traverse Tuple using Negative Indexing
('Java', 'Azure', 'AWS', 'Python', 14, 15, 16)
index : -1 | value : 16
index : -2 | value : 15
index : -3 | value : 14
index : -4 | value : Python
index : -5 | value : AWS
index : -6 | value : Azure
index : -7 | value : Java
From the program output, all elements of the tuple are printed based on their negative index position. Elements were iterated from right to left. Element at index -1
was printed and then subsequent elements towards left.
Error – Index Out of Range
Positive index and negative index, both are assigned as per the number of elements present in the tuple. If we pass an out of range index position for the tuple, then an error will be raised.
Error with Positive Index
# Error out of range - positive index
shbytes_tuple = ("Java", "Azure", "AWS", "Python", 14, 15, 16)
print(shbytes_tuple)
print("element at index 7 - ", shbytes_tuple[7]) # passed out of range positive index
In this example program, the shbytes_tuple
has total 7 elements. Positive indexing (left to right) will be from index 0
to 6
. Index 7
is out of this range.
Output – Example Program – Error with Positive Index
Traceback (most recent call last):
File "D:-index-element-tuple.py", line 35, in <module>
print("element at index 7 - ", shbytes_tuple[7])
~~~~~~~~~~~~~^^^
IndexError: tuple index out of range
Index 7
is not present for the given tuple. So, an error is raised => IndexError: tuple index out of range
Error with Negative Index
# Error out of range - negative index
shbytes_tuple = ("Java", "Azure", "AWS", "Python", 14, 15, 16)
print(shbytes_tuple)
print("element at index -8 - ", shbytes_tuple[-8]) # passed out of range negative index
Total 7 elements are present in the given tuple. Negative indexing will be from -1 to -7. Index -8 is out of this range.
In this example program, total 7 elements are present in the shbytes_tuple
. Negative indexing (right to left) will be from -1
to -7
. Index -8
is out of this range.
Output – Example Program – Error with Negative Index
Traceback (most recent call last):
File "D:-index-element-tuple.py", line 39, in <module>
print("element at index -8 - ", shbytes_tuple[-8])
~~~~~~~~~~~~~^^^^
IndexError: tuple index out of range
Index -8
is not present for the given tuple. So, an error is raised => IndexError: tuple index out of range
Summary
In this tutorial we learned about how to access the elements within a tuple using both positive and negative indexing in Python. This explained about the concept of index positions, including how elements can be accessed from left to right with positive indexing and right to left with negative indexing. It also outline common mistakes one encounters with both positive and negative indices. The article shows programs on how to iterate through a tuple by using both approaches, including the potential errors encountered if the index is out of range than the length of the tuple.
Code snippets and programs related to Access Tuple Elements by Index in Python, can be accessed from GitHub Repository. This GitHub repository all contains programs related to other topics in Python tutorial.
Interview Questions & Answers
Q: What happens if access an out of range index in tuple?
If we attempt to access an index that doesn’t exist in the tuple (i.e., the index is greater than or equal to the length of the tuple), Python will raise an IndexError
.
mixed_tuple = (12, 'shbytes', 33.14)
print(mixed_tuple[3]) # Raises IndexError: tuple index out of range
Q: How can we access a range of elements in a tuple?
To access a range of elements in a tuple, we use tuple slicing. Slicing is done by specifying a start index and an end index separated by a colon :
. The start index is inclusive, and the end index is exclusive.
mixed_tuple = (12, 'shbytes', 33.14, "Python")
print(mixed_tuple[1:4]) # slicing elements: ('shbytes', 33.14, 'Python')
Q: What are some practical use cases for accessing tuple elements by index?
- Data unpacking – Tuples are often used to store multiple pieces of related data (e.g., coordinates, RGB color values). Accessing tuple elements by index allows you to extract specific data.
- Working with function returns – Functions can return multiple values as a tuple. We can access each return value by index.
- Database queries – Tuples are used to represent rows in a database query result, where accessing elements by index corresponds to accessing column values.
Q: What is the difference between accessing elements in a tuple and a list?
The syntax for accessing elements in both a tuple and a list is identical, as both use zero-based indexing.
The key difference lies in the mutability of the data structure. Lists are mutable, allowing modification, addition, or removal of elements, while tuples are immutable, meaning their contents cannot be changed once created.
Related Topics
- Understand the Index Method in Python Tuples: Use Cases, Limitations, and ExamplesElements in the tuple are stored based on their index positions. Python provides the index(arg) method to determine the index position of an element in a tuple. In previous article Access tuple elements by index in Python, we learned about accessing the tuple element at the given index position. In this article we will learn…
- Tuples in Python: Operations, Definition & ExamplesTuples in Python Python tuples is a sequence used to store elements (a collection of data). Similar to arrays and lists, tuples also store elements based on their index and can be accessed based on the index position. Understanding Python Tuples: A Core Datatype Tuples are core data types in Python, alongside list, set, and…
- Slicing of Tuple in Python: Methods, Examples & Interview QuestionsSlicing of a tuple means extracting a part (or slice) of the tuple. In Python, tuple slicing can be done in two primary ways: In previous articles, we learned about Access Tuple Elements by Index in Python, How to Change Elements in a Tuple in Python and How to Remove Elements from a Tuple in Python.…
- Python Tuple – Practice Program 2In previous articles, we learned about various functions and operations that we can perform on Tuples in Python. In this article we will work on practice programs related to Python Tuples. Program – Problem Statement We will be given two tuples with number elements. We need to create all unique pair combinations from these two…
- Python Tuple – Practice Program 1In previous articles, we learned about various functions and operations that we can perform on Tuples in Python. In this article we will work on practice programs related to Python Tuples. Program – Problem Statement We will be given a nested tuple whose elements will also be tuple. Each tuple element can have multiple numbers…