Why Learn Python Programming Language?
Python is an interpreted, high level, general-purpose programming language. It is not a straight compiled language like C++ & Java.
Python is also a dynamic typed language with easy syntax. In Python datatype declaration of a variable is not required before using that variable.
Python supports procedural, object oriented & functional programming paradigms.
- Procedural means it supports program workflow control using loops and control statements.
- Object Oriented Programming (OOPs) features like Class, Inheritance, Polymorphism, Encapsulation and Abstraction.
- Functional programming paradigms, where sequential program workflow can be written using functions, conditional expressions and recursion.
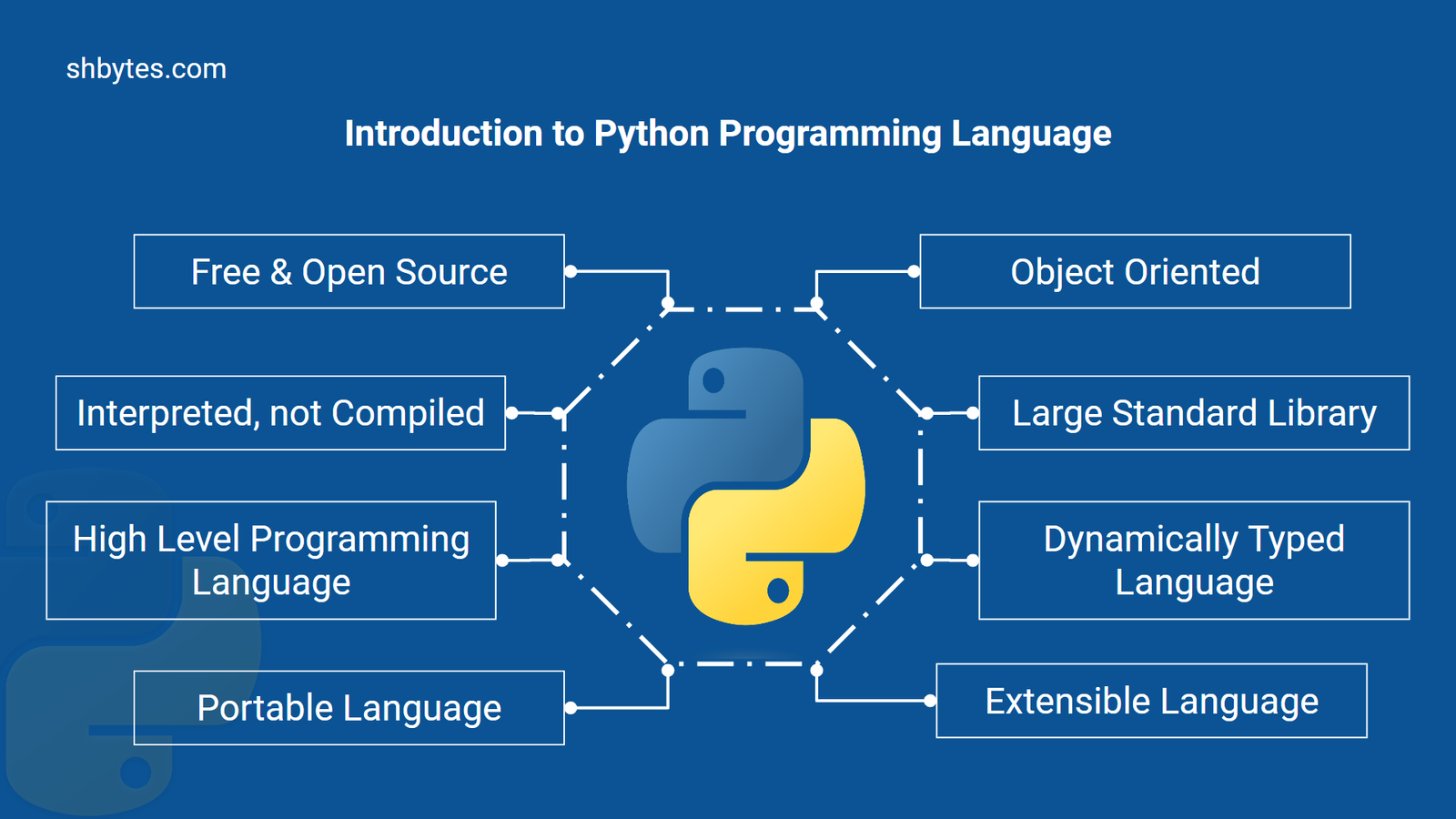
Origin of Python
Python was designed and created by Guido van Rossum and first released in 1991. It was designed as a successor to the ABC programming language with a focus on simplicity and readability. Van Rossum aimed to develop a language that was both powerful and easy to learn, incorporating ideas from other languages like C, Java, and Perl, while improving upon their complexities.
Python quickly gained popularity for its clean syntax, dynamic typing, and extensive libraries, making it ideal for both beginners and experienced developers. The language has since evolved into one of the most widely used programming languages worldwide, powering applications in web development, automation, data analysis, artificial intelligence, and more.
Python Official Website
Python’s official website – https://www.python.org is comprehensive resource and primary hub for the Python programming language. It offers:
- The latest Python releases for various platforms like Windows, Linux, MacOS.
- Wide range of resources including detailed documentation for each version, tutorials, and guides for developers of all skill levels.
- Access to Python’s extensive standard library, information on community events, and links to official packages and third-party modules.
- It’s a go-to platform for downloading Python, learning about its updates, and engaging with the Python developer community.
- Access to Python community forums, events, and discussions.
Key Programming Paradigms in Python
Python supports several key programming paradigms, making it versatile for various types of development:
- Object-Oriented Programming (OOP) – Python allows to create and manage classes and objects, supporting encapsulation, inheritance, and polymorphism. This paradigm is helpful for structuring complex software with reusable components.
- Procedural Programming – Python supports the procedural paradigm, where programs are organized into functions and procedures. This approach is often used for simpler scripts and applications where a clear step-by-step process is followed.
- Functional Programming – Python also supports functional programming, focusing on the use of functions as first-class objects. It includes features like lambda functions, higher-order functions, map, filter, and reduce, emphasizing immutability and pure functions.
- Imperative Programming – Python allows for imperative programming, where the code specifies the exact steps that the computer must take to reach a desired state, with a focus on commands and changes to program state.
- Meta-programming – Python supports meta-programming, which allows code to manipulate itself or other code. This is facilitated through dynamic features like decorators and meta-classes.
- Aspect-Oriented Programming (AOP) – While not native to Python, AOP can be achieved using decorators and meta-programming techniques to separate cross-cutting concerns, like logging or authentication.
These paradigms make Python flexible for different use cases, from simple automation scripts to complex, scalable systems.
Core Principles of Python: The Zen of Python
Python programming language was designed & built with core principals summarized in document “The Zen of Python“.
Core principals includes some popular aphorisms or memorable general truths.
- Beautiful is better than ugly
Python avoids use of multiple different characters like semicolon (;), curly brackets ({}) to separate one line or block of code from another. Instead, Python uses the indentation to signify a block of code inside the other statements.
- Explicit is better than implicit
Python was designed to have a small core implicitly built, which is required for working of a programming language and its features.
But it has a large explicitly & extensible built libraries which can be used as per the functional requirement of applications. Python has many libraries like Pandas, NumPy, SciPy, Matplotlib, Scikit-learn etc. These libraries are very popular for Machine Learning and Data Analytics.
- Simple is better than complex
Python is very easy to use, easy to learn and work. The lines of code required to complete a functionality in Python, is much less as compared to other programming languages. Example for Java and Python:
JAVA
Class HelloWorld {
public static void main(String args[]) {
System.out.println("Hello Shbytes");
}
}
Python
print "Hello Shbytes"
- Readability counts
Python code seems simple, easy and a smaller number of lines. It makes it easily readable and understandable.
- Complex is better than complicated
This principal is related to Python usage for development. Complex and complicated sounds similar and seems alike, but they are different. Complex includes wrapper functions in a single command or functionality, but complicated writes every line to achieve a functionality.
Complicated
counter = 0
while counter < 5:
print counter
counter += 1
Complex
for i in xrange(5):
print i
In this example, in complicated every line of code is explained and we can understand it easily, but most of task need to perform manually. Like assigning counter to zero and then comparing counter to less than 5 is not required. In complex, it’s not easy to understand at first glance, but after understanding the working of function xrange(), it is obvious and becomes easy to understand.
- Errors should never pass silently, unless explicitly silenced
Python does not allow errors to pass silently. Python always throw(s) errors & disrupt the program execution workflow, until unless errors are explicitly defined to be silenced.
- Now is better than never
Python tries to do things sooner. Also, Python is simple & easy to learn, and we should also learn it now.
- If the implementation is easy to explain, it may be a good idea
Python follows implementation of functionality to be easy weather it requires some extra variables or slightly more memory. Complicated functional implementation becomes blocker in later stages.
These core principals give us some understanding for Python programming language.
Key Characteristics of Python
There are multiple features provided by Python Programming Language.
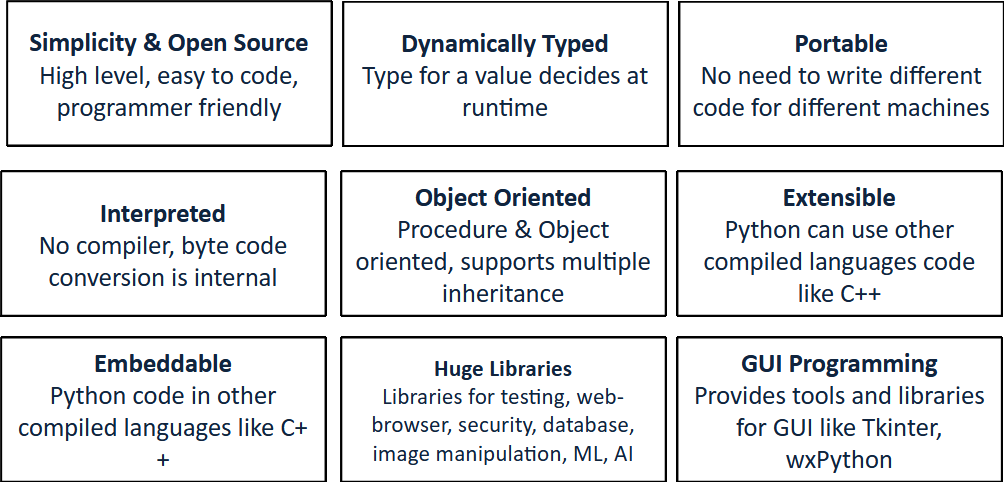
Python is an open source programming language
Python is an open source programming language. Open source means its source code is available and can be accessed from their official website https://www.python.org/downloads/source/. Python’s latest releases for multiple platforms are freely available from their official website https://www.python.org/downloads/. As an open source programming language we can contribute in the development of Python and can also use it for development of third party or commercial applications.
Python is a simple high-level programming language
High level programming language means Python is agnostic to underlying system architecture. To write programs in Python, we do not need to remember the underlying system architecture, nor we must manage the memory or data storage into the memory.
The lines of code required to complete a functionality in Python, is much less as compared to other programming languages, that why it’s simple, easy to code and programmer friendly as well.
Python is a dynamically typed language
Dynamically types language means declaration of variable with predefined datatype is not required. But the data type like (number, string, list, etc.) of variables are determined at run time based on the values of variables. This feature helps in many scenarios of data analysis, where the datatype of data is not known beforehand.
Python is a portable language
Portable language means, Python code written on one platform can be used on other platforms and different machines without any change.
For example, for a Python program written on Windows, this same program, without any change can be used on other platforms like Linux, Unix, and Mac OS. This feature is very helpful to create an application to work on multiple platforms.
Python is an interpreted language
Python code is executed line by line. Like C, C++ and Java there is no need to keep the code compiled before its execution. This makes easier to debug the code.
Source code of Python is converted to its immediate form bytecode. This also saves compilation and building of application time, which helps in speedup the development and deployment of applications.
Python is a procedural and Object-Oriented Programming language
Python supports concepts of Object-Oriented Programming like Class, Inheritance, Polymorphism, Encapsulation and Abstraction.
This is a key feature, which makes Python a competitive programming language for C++, Java and C#. This feature also makes Python to be used for development of web-applications and their related frameworks.
Python also supports concepts of multiple inheritance.
Python is also an extensible language
Extensible language means, we can use Python to write code for other programming language like C++.
Python is an embeddable language.
Embeddable language means, we can write the Python code with other programming languages like C, C++ or Java and same Python code can be compiled with the same programming language.
Python has a large standard libraries and frameworks
Large standard libraries and frameworks provides a rich set of modules and functions.
Libraries provides already written and tested code, so we don’t have to write everything. This makes development faster and more focused on the application functionality.
There are many popular libraries and frameworks for analysis, regular expressions, unit-testing, web development, web-browser, security, database, image manipulation, machine learning, data analytics and artificial intelligence etc.
Some of the popular libraries of Python are Pandas, NumPy, SciPy, Matplotlib, Scikit-learn etc
Python also provide GUI (Graphical User Interface)
Python also provides GUI (Graphical User Interface) programming tools and libraries like Tkinter, wxPython.
Why Python – Use of Python in Industrial Applications
Python, due to its versatility, ease of use, and powerful libraries is widely used across IT industry. Here are some key uses of Python in various sectors:
- Web Development – Python frameworks like Django and Flask are popular for building web applications due to their flexibility and scalability.
- Data Science & Analytics – Python is a favorite for data analysis, visualization, and machine learning. Libraries like Pandas, NumPy, Matplotlib, and Scikit-learn make it more useful for data professionals.
- Artificial Intelligence & Machine Learning – Python’s simplicity allows for fast prototyping in AI/ML projects. Tools like TensorFlow, Keras, and PyTorch are widely used in research and industry.
- Automation & Scripting – Python is used to automate repetitive tasks, from simple file operations to complex server management, with tools like Selenium and Automation Anywhere.
- Fintech & Quantitative Finance – Python helps in building financial models, risk management tools, and real-time trading systems, using libraries like QuantLib.
- Cloud Computing – With the growth of cloud services, Python is often used for managing cloud infrastructure, writing server-less applications, and interacting with cloud providers like AWS, Azure and GCP.
- Cybersecurity – Python is used for writing security tools, automating malware analysis, penetration testing, and network security auditing, using libraries like Scapy and Impacket.
- Game Development – Python’s Pygame library is often used in 2D game development and for prototyping game logic in larger projects.
- Embedded Systems – Python is gaining traction in the development of embedded systems, especially with tools like MicroPython and CircuitPython.
- Healthcare & Bioinformatics – Python is used for data analysis in healthcare, building applications for patient care monitoring, genomics, and bioinformatics.
Python’s extensive library support, ease of learning, and ability to integrate with other technologies make it a go-to language for numerous industrial applications.
Summary
Python is a versatile and beginner-friendly programming language known for its simplicity and readability, making it an ideal choice for both new and experienced developers. Created by Guido van Rossum in 1991, Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming. Its extensive standard library, along with a wide range of third-party modules, allows developers to efficiently build web applications, automate tasks, analyze data, and more. Python’s growing popularity is also driven by its vibrant community and strong applicability in fields like data science, artificial intelligence, and machine learning.
In this article we learned about various topics on Introduction to Python Programming Language. We discussed on following topics:
- Why Learn Python Programming Language?
- Origin of Python
- Python Official Website
- Key Programming Paradigms in Python
- Core Principles of Python: The Zen of Python
- Key Characteristics of Python
- Why Python – Use of Python in Industrial Applications
Test Your Knowledge: Practice Quiz
Related Topics
- What Are Comments and Docstring in Python (With Programs)?Before we start learning about Comments and Docstring in Python and its concepts, we should setup our Python Integrated Development Environment (IDE) on our local machine. Read through the following articles to learn more about What is an IDE, Popular…
- Popular IDEs for Python in 2024 – Best Python Development EnvironmentsIDEs are very important tool (application) for the software development. Working with good IDE improves the performance and working capability of a software developer. That is why it’s important to choose a good IDE for software development. Different developers choose…
- What is an IDE (Integrated Development Environment) – A Complete Guide for DevelopersWhat is an IDE (Integrated Development Environment) An Integrated Development Environment (IDE) is a robust software tool (application) that helps programmers and developers write code more efficiently. It combines multiple features such as code editing, building, testing, and packaging into…
- How to Install Python 3 on Ubuntu: A Comprehensive GuideTo start developing applications using Python 3, we need to install Python 3 on our machines. Article Python 3 Installation on Windows provides comprehensive details about installation of Python 3 on Windows 10 or Windows 11. Linux-based production environments are…
- Python 3 Installation on WindowsPython 3 Installation on Windows 10/11: Complete Step-by-Step Guide To start developing applications using Python 3, we need to install Python 3 on our machine. This guide will show you how to install Python 3 on Windows 10 or Windows…