Array is a most fundamental and useful data structure in Python Programming Language. Python programming provides the array
module, which allows to create arrays. Array
is a sequential collection of elements of homogeneous datatype that are stored at contiguous memory locations. Array
in Python are more memory-efficient than lists. Python Arrays are very helpful to store large amounts of homogeneous data (data of the same datatype).
In previous articles, we learned about other collection datatypes in Python. Read following articles to learn more about List, Tuple, Set and Dictionary:
- Lists in Python – Quickstart
- Tuple in Python – Quickstart
- Set in Python – Quickstart
- Dictionary in Python – Quickstart
Lets understand about various key properties of Array in Python.
Properties of Array in Python
Python arrays has specific properties which makes them more efficient that lists. These properties include type constraint, memory efficiency, fixed size, and more.
- Homogeneous Data – Python arrays support only single datatype elements. All elements stored in an array, should be of specific datatype (e.g., integers, floating-point numbers, characters).
- Type Codes – Array elements datatype is specified by the Type Codes. For example,
'i'
for integers and'f'
for floating-point numbers. - Memory Optimized Storage – Arrays in Python are more memory-efficient than lists. This is very helpful in handling of large amounts of data. Because of uniform datatype elements in array, Python can allocate memory more efficiently, reducing overhead.
- Contiguous Memory Layout – Array elements are stored sequentially in memory. This helps to improve data cache and performance of applications.
- Fixed Size – Specific size (number of elements) is defined when an array object is created and memory allocation is also based on the defined number of elements.
- Dynamic Resize – Append or insert operations are allowed on array object, but this operation will involve reallocation of memory. This dynamic resize reduces performance of arrays and make them less efficient than lists.
- Indexing – Arrays are indexed and its elements can be accessed based on their index positions. Arrays can achieve O(1) time complexity for accessing elements by index.
- Basic Operations: Python arrays support a range of operations similar to lists, such as slicing, concatenation, and iteration except some of the advanced functionalities provided by lists.
- Immutable Type Code – Arrays type code is immutable and cannot be changed. It means, once an array is created with a specific type code, we cannot change the type code for this array. We can delete this array and can create a new array with different type code.
- 1D Arrays – By default, Python built-in array module supports only one-dimensional arrays. For multidimensional arrays support we can use Python library NumPy.
- Interoperability with C – Python arrays can easily interacting with C libraries can be very helpful to pass data to and from C code.
Index of an element in Array
Elements in the array
are stored sequentially based on their index positions. Index assignment to array
elements is similar to index assigned in List elements.
Two ways in which index can be assigned to array elements:
- Index from left to right (Positive Indexing)
- Index from right to left (Negative Indexing)
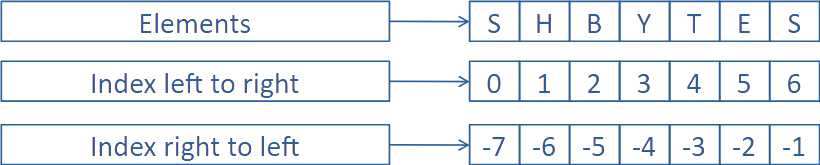
Index from left to right (Positive Indexing)
Index of an element in the array
starts from left to right. First element from left is assigned index 0 and then index increased one at a time in the right direction. The right most element i.e. last element of array will have index = number of elements in array -1. This indexing from left to right also known as Positive Indexing.
As shown in the above diagram, Array elements are [‘S’, ‘H’, ‘B’, ‘Y’, ‘T’, ‘E’, ‘S’]
. Total number of elements in the array are 7
. First element i.e. ‘S’ will have index 0 and then index of each element towards right will increase by 1. Last element i.e. ‘S’ again will have index 6.
Index from right to left (Negative Indexing)
Index of an element in array can also starts from right to left. Right most element i.e. last element is assigned index -1 and then index decreased one-by-one in the left direction. The left most element i.e. first element of the array will have index = negative of number of element in array. This indexing from right to left also known as Negative Indexing.
As shown in the above diagram, Array elements are [‘S’, ‘H’, ‘B’, ‘Y’, ‘T’, ‘E’, ‘S’]
. Total number of elements in the array are 7. Last element i.e. ‘S’ will have index -1 and then index for each elements towards left will decrease by 1. First element i.e. ‘S’ again will have index -7.
Representing 1-D, 2-D and 3-D Arrays
By default, Python built-in array
module supports only one-dimensional (1-D) arrays. For multidimensional arrays (2-D and 3-D) support we can use Python library NumPy. Here we are just giving an example, how one-dimensional (1-D), two-dimensional (2-D) and three-dimensional (3-D) arrays can be represented.

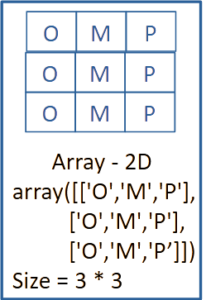
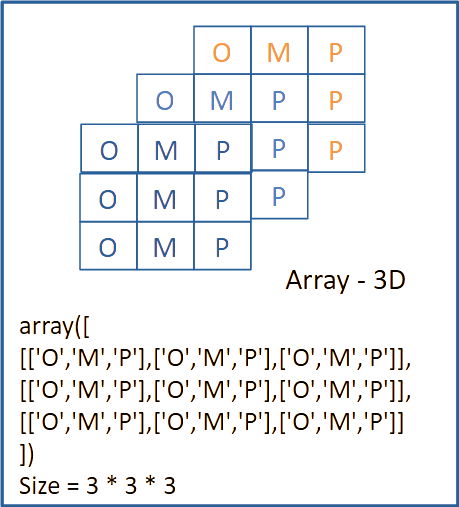
- One Dimensional (1-D) array can be represented as
array(['O','M','P’])
, which is ofsize = 1 * 3
=> 1 row and 3 columns. - Two Dimensional (2-D) array is an array of 1-D arrays and can be represented as
array([['O','M','P'],['O','M','P'],['O','M','P’]])
, which is ofsize = 3 * 3
=> 3 rows and 3 columns. - Three Dimensional (3-D) array is an array of 2-D arrays and can be represented as
array([[['O','M','P'],['O','M','P'],['O','M','P']],[['O','M','P'],['O','M','P'],['O','M','P']],[['O','M','P'],['O','M','P'],['O','M','P']]])
, which is ofsize = 3 * 3 * 3
=> 3 rows, 3 columns and 3 stacks.
Array Type Codes
Array elements datatype is specified by the Type Codes. An array in Python can only store elements of specified type code. Array type codes are single-character and define the type of elements. Below is a list of common array type codes:
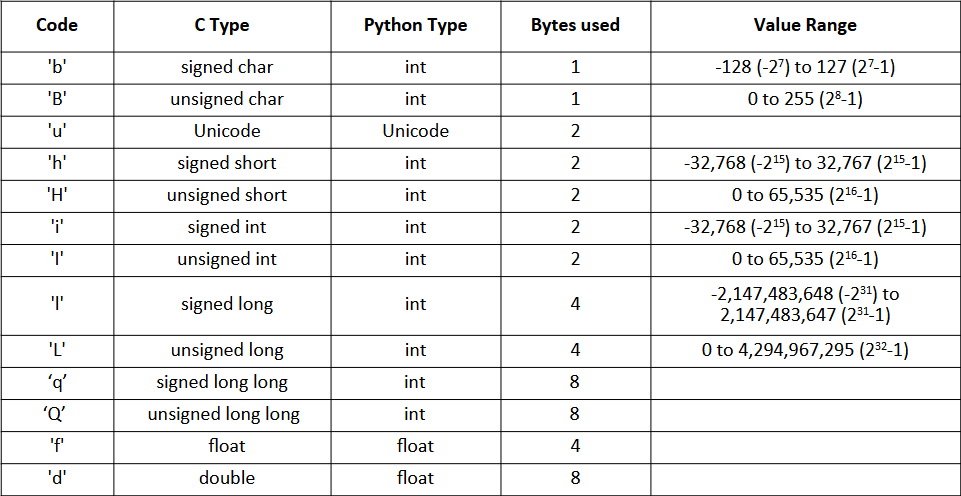
Create array in Python
Python provides the array
module, which allows to create arrays. To create an array in Python, we need to import the array
module and then we can user the array()
method to create an array object. Syntax of array()
method:
array_object = array.array(typecode, [initializer])
typecode
(mandatory) – Type code is mandatory field. An array in Python can only store elements of specified type code. For example,'i'
for integers and'f'
for floating-point numbers etc.initializer
(Optional) – Thisinitializer
can be a collection datatype like list, tuple, or any iterable to define the initial values for the array. Ifinitializer
is provided, then array will be initialized with these values else an empty array will be created.array_object.typecode
– This can print the type code used to create an array- Note – Numbers in the array should be within the given range for defined type code.
Create array of typecodes
Almost all type codes support the integer numbers. But numbers we want to store in the array should be within the given range for defined type code.
signed and unsigned char array
‘b’ for signed char (1 byte) (range => -128 to 127) array and ‘B’ for unsigned char (1 byte) (range => 0 to 255) array.
import array as array # import array module
signed_char_array = array.array("b", [10, -20, 30, -40]) # typecode 'b' signed char
print(signed_char_array)
print("signed char type", signed_char_array.typecode)
unsigned_char_array = array.array("B", [10, 20, 30, 40]) # typecode 'B' unsigned char
print(unsigned_char_array)
print("unsigned char type", unsigned_char_array.typecode)
Program Output
array('b', [10, -20, 30, -40])
signed char type b
array('B', [10, 20, 30, 40])
unsigned char type B
OverflowError – Number out of typecode range
import array as array # import array module
unsigned_char_array = array.array("B", [10, 20, 30, 4660]) # typecode 'B' unsigned char
# Program Output
# Traceback (most recent call last):
# File "D:\array-typecode-method.py", line 16, in <module>
# unsigned_char_array = array.array("B", [10, 20, 30, 4660])
# ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
# OverflowError: unsigned byte integer is greater than maximum
import array as array # import array module
unsigned_char_array = array.array("B", [10, 20, 30, -4660]) # typecode 'B' unsigned char
# Program Output
# Traceback (most recent call last):
# File "D:\array-typecode-method.py", line 16, in <module>
# unsigned_char_array = array.array("B", [10, 20, 30, -4660])
# ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
# OverflowError: unsigned byte integer is less than minimum
signed and unsigned short array
‘h’ for signed short (2 byte) (range => -32768 to 32737) array and ‘H’ for unsigned short (2 byte) (range => 0 to 65535) array.
‘i’ for signed int (2 byte) (range => -32768 to 32737) array and ‘I’ for unsigned int (2 byte) (range => 0 to 65535) array.
import array as array # import array module
signed_short_array = array.array("h", [2330, -2230, 6730, -8740]) # typecode 'h' signed short
print(signed_short_array)
print("signed char type", signed_short_array.typecode)
unsigned_short_array = array.array("H", [1670, 2980, 9860, 49660]) # typecode 'H' unsigned short
print(unsigned_short_array)
print("unsigned char type", unsigned_short_array.typecode)
Program Output
array('h', [2330, -2230, 6730, -8740])
signed char type h
array('H', [1670, 2980, 9860, 49660])
unsigned char type H
signed and unsigned long array
‘l’ for signed long (4 bytes) (range => -2147483648 to 2147483647) array and ‘L’ for unsigned long (2 bytes) (range => 0 to 4294967295) array.
import array as array # import array module
signed_long_array = array.array("l", [-341000, 452000, -8783000, 8774000]) # typecode 'l' signed long
print(signed_long_array)
print("signed long type", signed_long_array.typecode)
unsigned_long_array = array.array("L", [22321000, 76542000, 878543000, 96544000]) # typecode 'L' unsigned long
print(unsigned_long_array)
print("unsigned long type", unsigned_long_array.typecode)
Program Output
array('l', [-341000, 452000, -8783000, 8774000])
signed long type l
array('L', [22321000, 76542000, 878543000, 96544000])
unsigned long type L
Unicode array
‘u’ for unicode (2 bytes) array.
# array of Unicode type
print("array of Unicode type")
import array as array # import array module
unicode_array = array.array("u", ['o', 'm', 'p', 'r']) # typecode 'u' unicode
print(unicode_array)
print("Unicode type", unicode_array.typecode)
Program Output
array of Unicode type
array('u', 'ompr') # output for an unicode array
Unicode type u
Float and empty array
‘f’ for float (4 bytes) array and ‘d’ for double (8 bytes) array.
import array as array # import array module
empty_array = array.array('f') # empty array, that can store float numbers
print(empty_array)
float_array = array.array('f', (5.1, 42.2, 37.38)) # typecode f, float numbers
print(float_array)
Program Output
array('f')
array('f', [5.099999904632568, 42.20000076293945, 37.380001068115234])
Summary
In this article we explored about arrays in Python. Following topics were discussed:
- Properties of Array in Python
- Index of an element in Array
- Index from left to right (Positive Indexing)
- Index from right to left (Negative Indexing)
- Representing 1-D, 2-D and 3-D Arrays
- Array Type Codes
- Create array in Python
- Create array of typecodes
This was very informative. I appreciate the clarity and depth.