List is a sequence data type used to store elements (collection of data). Lists are similar to Arrays but have a dynamic size, meaning the list size can increase or decrease as elements are added or removed.
A list is a core data type in Python, along with Tuple, Set and Dictionary. Lists in Python are of <class 'list'>
and are mutable objects, meaning we can perform add (append), update (change), and delete (remove) operations on list objects.
In this article, we will learn about different methods to create List objects in Python.
Methods to Create List in Python
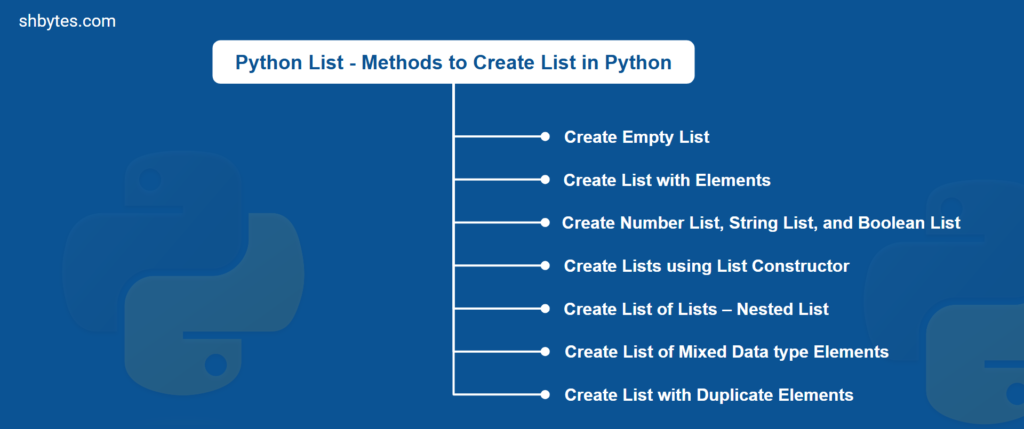
List objects in Python can be created in multiple ways. We can declare and initialize a list simultaneously.
- Create Empty List
- Create List with Elements
- Create Number List, String List, and Boolean List
- Create Lists using List Constructor
- Create List of Lists – Nested List
- Create List of Mixed Datatype Elements
- Create List with Duplicate Elements
Create Empty List
An empty list can be created using square brackets without passing any elements within the square brackets.
# empty list creation with brackets
empty_list = []
print(empty_list)
print(type(empty_list))
In this example program, we have created an empty list using square brackets referenced by a variable name empty_list
.
Output – Example Program – Create Empty List
[]
<class 'list'>
The output shows an empty list with empty square brackets, and the data type of the object is <class 'list'>
.
Create List with Elements
A list with elements can be created by passing comma-separated elements within square brackets.
# String list creation with brackets
string_list = ['Python', 'Java', 'AWS', 'Azure'] # list of string
print(string_list)
print(type(string_list))
In this program, a list with string elements is created, referenced by the variable name string_list
.
Output – Example Program – Create List with Elements
['Python', 'Java', 'AWS', 'Azure']
<class 'list'>
Create Number List and Boolean List
Using the same method as used to create list with string elements, we can create lists with number elements and boolean elements.
# Number list creation with brackets
num_list = [30, 40, 50, 60] # list of numbers
print(num_list)
print(type(num_list))
# Boolean list creation with brackets
boolean_list = [False, True, True, True] # list of boolean
print(boolean_list)
print(type(boolean_list))
In this example program, two lists are created. num_list
is a list with number elements, while boolean_list
contains boolean values.
Output – Example Program – Create Number List and Boolean List
[30, 40, 50, 60]
<class 'list'>
[False, True, True, True]
<class 'list'>
In the output list number elements and boolean elements within square bracket are shown.
Create Lists Using List Constructor
We can also create a list using the list constructor.
# list creation with list constructor
list_m = list(('Python', 'Java', 'AWS', 'Shbytes'))
print(list_m)
print(type(list_m))
In this example, a list is created using the list constructor, which takes a single argument that combines all elements within parentheses.
Output – Example Program – Create Lists Using List Constructor
['Python', 'Java', 'AWS', 'Shbytes']
<class 'list'>
In the output we have list elements within square bracket.
Create List of Lists – Nested List
We can store multiple lists within a list itself. This type of list is called a nested list.
# list of lists
nested_list = [['C', 2], [2.4, 3], ['AWS', 6], 4]
print(nested_list)
print(type(nested_list))
In the nested list, each element is also a list. We have created a nested list referenced with variable name nested_list
.
Output – Example Program – Create List of Lists – Nested List
[['C', 2], [2.4, 3], ['AWS', 6], 4]
<class 'list'>
In output we got elements of the nested list and type of object.
Create List of Mixed Datatype Elements
Unlike nested lists, you can store multiple data types within a single list, such as numbers, boolean, strings, and other collections. In this program we will create a mixed datatype elements in the list.
# list of mixed collections
mixed_list = [1.13, 5, "shbytes", {'Azure': 1}, ['AWS', 2]] # contains float, integer, string, dictionary and list elements
print(mixed_list)
print(type(mixed_list))
In this program, mixed_list
contains a float, integer, string, dictionary, and list elements.
Output – Example Program – Create List of Mixed Datatype Elements
[1.13, 5, 'shbytes', {'Azure': 1}, ['AWS', 2]]
<class 'list'>
In output we have print the list and the type of object.
Create List with Duplicate Elements
Lists can contain duplicate elements, unlike sets, which do not support duplicates.
# list allows duplicate elements
duplicate_element_list = ["Python", "Java", "AWS", "Azure", "AWS"]
print(duplicate_element_list)
print(type(duplicate_element_list))
We have created a list object which stores duplicate elements. In this list, the string element “AWS” appears twice.
Output – Example Program – Create List with Duplicate Elements
['Python', 'Java', 'AWS', 'Azure', 'AWS']
<class 'list'>
Summary
In this article, we covered multiple ways to create lists in Python, including empty lists, lists with elements, number and boolean lists, lists using the constructor, nested lists, mixed data type lists, and lists with duplicate elements. These techniques offer flexibility and are fundamental to working with data in Python.
Code snippets and programs related to Create list in Python, can be accessed from GitHub Repository. This GitHub repository all contains programs related to other topics in Python tutorial.
Interview Questions & Answers
Q: How a list can be initialized with a specific size and default values?
A list can be initialized with a specific size and default values using list multiplication.
size = 5
default_value = 0
initialized_list = [default_value] * size
This will create a list initialized_list
with 5 elements, all initialized to 0
=> [0, 0, 0, 0, 0]
Q: How a list can be created for numbers from 1 to 10?
To create a list of numbers from 1 to 10, we can use range()
function inside list()
or by list comprehension.
- Using
range()
function =>numbers = list(range(1, 11))
- Using comprehension =>
numbers = [x for x in range(1, 11)]
list("shbytes")
?
Q: What will be the output list from list("shbytes")
will create a list where each character of the string “shbytes” becomes an element in the list.
list("shbytes")
=> [‘s’, ‘h’, ‘b’, ‘y’, ‘t’, ‘e’, ‘s’]
Q: How can we create a copy of a list in Python?
We can create a copy of a list using slicing, list()
, copy()
or deepcopy()
methods.
- Using slicing =>
copied_list = original_list[:]
list()
ORcopy()
=>copied_list = list(original_list)
ORcopied_list = original_list.copy()
deepcopy()
=>copied_list = copy.deepcopy(original_list)
, in this case we need to importcopy
module.
Q: Create a list with n elements, each initialized to None?
none_list = [None] * n
=> This will create none_list
with elements [None, None, None, None, None]
.
Q: Difference between a shallow copy and a deep copy of a list. How do you create a deep copy?
Shallow copy can be created with either of slicing, list()
, copy()
methods. For deep copy we can use deepcopy(
) method from copy
module.
shallow_copy_list = original_list[:]
shallow_copy_list = list(original_list)
Using copy
module. Need to import copy
module first.
shallow_copy_list = original_list.copy()
deep_copy_list = copy.deepcopy(original_list)
Test Your Knowledge: Practice Quiz
Related Topics
- Count & Sort Methods in Python Lists: Tutorial with ExamplesThe count() & Sort() methods are built-in methods in Python lists, useful for counting occurrences of elements and sorting of elements in the list. Lists in Python can store duplicate elements and elements of various data types, making these methods essential for data handling. In previous article, we learned about Loops and List Comprehension in Python.…
- Loops and List Comprehension in Python: A Complete GuideLooping is a process to repeat the same task multiple times. It provides sequential access to elements in the list. Comprehension offers a shorter syntax to iterate (loop) through list elements. In previous article, we learned about How to Unpack List in Python. In this article we will learn about Python list loops and List…
- How to Unpack List in Python – Packing and Unpacking in Python (with Programs)Packing & unpacking are two processes that we can perform on lists in Python. We have seen the packing process in our previous article Concatenate Lists in Python, where we used packing to concatenate multiple list elements into a single list. In this article, we will learn in depth about How to Unpack a List…
- Concatenate Lists in Python – Complete Guide to List Concatenation MethodsConcatenation of lists in Python refers to merging of elements from two or more lists into a single list. Python provides a variety of methods for list concatenation, allowing flexibility based on the requirements of each task. For all topics related to lists, check out the Lists in Python: A Comprehensive Guide guide. In the…
- Introduction to List Slicing in Python – Methods, Syntax, and ExamplesList slicing in Python or slicing of list in Python means extracting a part (or slice) of the list. Slicing of list uses the index position of elements in the list. Slicing can be done using either of two methods: In previous articles, we learned about accessing list elements by index, using the list index method to…