A tuple is a sequence datatype used to store elements (a collection of data). Tuples in Python are immutable objects, meaning we cannot perform add (append), update (change), or delete (remove) operations on tuple objects. The size of tuple objects cannot be increased or decreased because we cannot add or remove elements from the tuple.
Tuple elements are indexed, and we can access the tuple objects similar to lists. A tuple is one of the core data types in Python along with lists, sets, and dictionaries. Tuples in Python are of the type <class ‘tuple’>
.
In this article, we will learn about different methods to create Tuple in Python.
Methods to Create Tuple
Tuple objects in Python can be created in multiple ways. We can declare and initialize the tuple simultaneously.
- Create Empty Tuple
- Create Tuple with Elements
- Create String and Boolean Tuple
- Create Tuple without Parentheses
- Create Tuple using Tuple Constructor
- Create Tuple of Tuples – Nested Tuple in Python
- Create Tuple of Mixed Datatype Elements
- Create Tuple with Duplicate Elements
- Create Tuple with Single Element
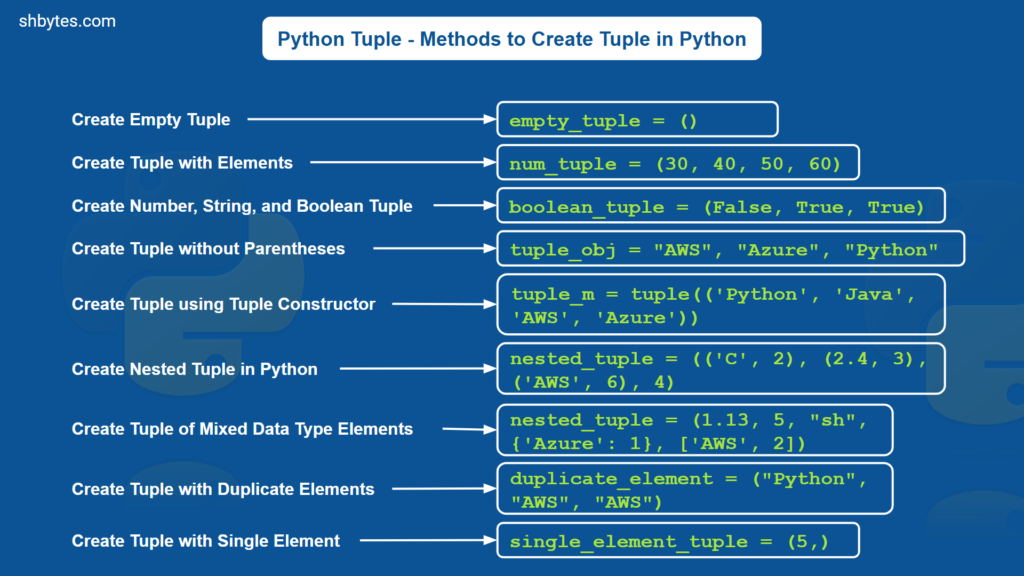
Create Empty Tuple
An empty tuple object can be created with parentheses and by not passing any element within the parentheses.
# empty tuple creation with parentheses
empty_tuple = ()
print(empty_tuple)
print(type(empty_tuple))
# Output
# ()
# <class 'tuple'>
In the program above, we have created an empty tuple using parentheses referenced by the variable name empty_tuple
. From the output of this program, we got empty tuple shown with empty parenthesis and the datatype of the object is <class 'tuple'>
.
Create Tuple with Elements
A tuple with elements can be created by passing comma-separated elements within the parentheses.
# tuple creation with parentheses
num_tuple = (30, 40, 50, 60) # tuple creation with parenthesis, tuple of numbers
print(num_tuple)
print(type(num_tuple))
# Output
# (30, 40, 50, 60)
# <class 'tuple'>
Here, we create a tuple with numeric elements, referenced by the variable name num_tuple
.
Create String and Boolean Tuple
We can create tuples with string elements and boolean elements.
# tuple creation with parentheses
string_tuple = ('Python', 'Java', 'AWS', 'Azure') # tuple of string elements
print(string_tuple)
print(type(string_tuple))
# tuple creation with parentheses
boolean_tuple = (False, True, True) # tuple of boolean elements
print(boolean_tuple)
print(type(boolean_tuple))
Two tuple are created in the above program. One tuple with string elements and referenced by a variable name string_tuple
and second tuple with boolean value and referenced by a variable name boolean_tuple
.
Output – Example Program – Create String and Boolean Tuple
('Python', 'Java', 'AWS', 'Azure')
<class 'tuple'>
(False, True, True)
<class 'tuple'>
Create Tuple without Parenthesis
Tuples can also be created without parenthesis. While creating tuple, parenthesis are optional.
# tuple creation parentheses are optional
tuple_obj = "AWS", "Azure", "Python" # Create tuple without parenthesis
print(tuple_obj)
print(type(tuple_obj))
# Output
# ('AWS', 'Azure', 'Python')
# <class 'tuple'>
In this example program, the tuple object is created without parenthesis.
Create Tuple using Tuple Constructor
We can create a tuple using the tuple
constructor.
# tuple creation with tuple constructor
tuple_m = tuple(('Python', 'Java', 'AWS', 'Azure'))
print(tuple_m)
print(type(tuple_m))
# Output
# ('Python', 'Java', 'AWS', 'Azure')
# <class 'tuple'>
In this example program, we are creating a tuple using tuple constructor like tuple(('Python', 'Java', 'AWS', 'Azure'))
. The tuple constructor takes a single argument, with all the elements wrapped in the parenthesis. This creates a tuple from it. From the output of this program, we have tuple elements within parenthesis.
Create Tuple of Tuples – Nested Tuple in Python
We can store multiple tuple elements within another tuple, resulting in a nested tuple.
# Create Tuple of Tuples – Nested Tuple in Python
nested_tuple = (('C', 2), (2.4, 3), ('AWS', 6), 4)
print(nested_tuple)
print(type(nested_tuple))
# Output
# (('C', 2), (2.4, 3), ('AWS', 6), 4)
# <class 'tuple'>
In this example program, we are creating a nested tuple referenced by a variable nested_tuple
. Elements of this nested tuple are also a tuple (created using parenthesis). From the output, we got elements of the nested tuple and type of object.
Create Tuple of Mixed Datatype Elements
Tuples can store multiple different data types, such as numbers, booleans, strings, lists, sets, dictionaries, and more. In this program we will create a mixed datatype elements in the tuple.
# Tuple of Mixed Datatype Elements
nested_tuple = (1.13, 5, "omprosoft", {'Azure': 1}, ['AWS', 2])
print(nested_tuple)
print(type(nested_tuple))
# Output
# (1.13, 5, 'omprosoft', {'Azure': 1}, ['AWS', 2])
# <class 'tuple'>
Here, we create a tuple that includes various data types: float, integer, string, set, and list. From the output of this program, we have print the tuple elements and the type of object.
Create Tuple with Duplicate Elements
Tuple can be created with duplicate elements.
# Tuple with Duplicate Elements
duplicate_element_tuple = ("Python", "Java", "AWS", "Azure", "AWS")
print(duplicate_element_tuple)
print(type(duplicate_element_tuple))
# Output
# ('Python', 'Java', 'AWS', 'Azure', 'AWS')
# <class 'tuple'>
This program demonstrates the creation of a tuple containing duplicate elements. String element AWS
was stored two times in the tuple.
Create Tuple with Single Element
# tuple with single element
single_element_tuple = (5,)
print(single_element_tuple)
print(type(single_element_tuple))
# Output
# (5,)
# <class 'tuple'>
To create a tuple with a single element, we must include a trailing comma after the element. If we don’t include the comma, it will be considered as a list.
Summary
This article explains various methods to create tuples in Python, a sequence datatype that is immutable. Key methods include creating an empty tuple, tuples with elements, string and boolean tuples, and using the tuple constructor. It also covers creating nested tuples, tuples with mixed data types, and tuples with duplicate elements. Additionally, it shows how to create a tuple with a single element using a trailing comma.
Tuples are indexed and can be accessed similarly to lists, but once created they cannot be modified. This feature makes them essential for immutable data storage in Python.
Code snippets and programs related to Create Tuple in Python, can be accessed from GitHub Repository. This GitHub repository all contains programs related to other topics in Python tutorial.
Interview Questions & Answers
Q: What are the reasons to use tuple instead of a list in Python?
- Immutability – If we want that a collection of items should not change throughout the program, then a tuple is preferred because it is immutable.
- Performance – Tuples are immutable, this makes tuples faster and improves their performance compared to lists. Python optimizes tuple’s memory usage and access time.
- Hashability – Tuples can be used as keys in a dictionary or elements in a set because they are hashable (assuming all elements inside the tuple are also hashable).
- Safety – Tuples being immutable, prevents accidental modification of data, which can be especially important in large codebase.
Q: Can a tuple contain mutable objects? What happens if a mutable object inside a tuple is modified?
Yes, a tuple can contain mutable objects like lists or dictionaries. While the tuple itself is immutable, the mutable objects within it can be modified.
number_tuple = ([12, 22, 32], 24)
number_tuple[0].append(44) # list inside the tuple is modified
# Elements in number_tuple ([12, 22, 32, 44], 24)
In this case, the tuple structure remains unchanged, but list inside the tuple is modified.
Q: What are some use cases for tuples in Python?
- Return Multiple Values from a Function – Functions in Python can return multiple values as a tuple.
def get_coordinates():
return (10, 20)
latitude, longitude = get_coordinates()
- Store Heterogeneous Elements – Tuples are often used to store records or heterogeneous data elements where each element has a different type or meaning. Example –
mixed_tuple = (1.13, 5, "shbytes", {'Azure': 1}, ['AWS', 2])
- Dictionary Keys – Tuples can be used as keys in dictionaries because they are hashable. Example –
tuple_dict = {('a', 1): "value1", ('b', 2): "value2"}
- Immutability – Tuple are used, when we need to ensure that a collection of items cannot be modified.
Related Topics
- Access Tuple Elements by Index in Python: Positive and Negative Indexing ExplainedElements in the tuple are stored based on their index positions. Index assignment to tuple elements is similar to index assigned in Array elements. We can access tuple elements by index index positions. Let’s first understand how index positions are assigned to tuple elements. Read article, to learn more about How to Access List Elements…
- Append Elements to Tuple in Python: Methods, Errors, and ExamplesTuples are immutable datatypes in Python, meaning their elements cannot be modified directly. To append element to tuple, we must follow specific steps: convert the tuple into a list (mutable datatype), append the element to the list, and then convert the list back to a tuple. In previous article, we learned about Access tuple elements…
- Concatenate Tuples in Python: Methods, Examples, and ProgramsConcatenation of tuples in Python refers to merging elements from two or more tuples into a single tuple. In this article, we will explore multiple ways to concatenate tuples in Python, from using the + operator to advanced methods like itertools.chain() and packing & unpacking with *. In previous articles, we learned about Access Tuple Elements…
- Count & Sort methods in tuple – PythonCount & Sort are built-in methods with tuple in Python. Tuple in Python can store duplicate & multiple datatype elements. Follow quick-start guide, for all topics related to tuple in Python – Tuple in Python – Quickstart Count method in tuple Syntax of the count method: count_value = tuple.count(object) There are multiple scenarios in which…
- Create Tuple in Python: Methods, Examples, and ExplanationA tuple is a sequence datatype used to store elements (a collection of data). Tuples in Python are immutable objects, meaning we cannot perform add (append), update (change), or delete (remove) operations on tuple objects. The size of tuple objects cannot be increased or decreased because we cannot add or remove elements from the tuple.…