“Learn Python Programming Basics – Beginner to Master” is a comprehensive guide designed to take you from absolute beginner to proficient in Python programming. Starting with the fundamentals such as variables, data types, and control structures, the course progressively covers more advanced concepts like object-oriented programming, error handling, and file manipulation. By focusing on hands-on examples and practical applications, this resource equips learners with the skills needed to build real-world projects and master Python’s powerful features. Perfect for anyone eager to start a career in programming or enhance their technical skills!
In today’s time Python is an important programming language. Python is a core programming language for Data Science, Machine Learning and Artificial Intelligence. That is why learning Python language has become important for software students and professionals. From this Python tutorial for beginners, we can learn Python basics used for data science and machine learning.
Lets learn all the topics covered in this comprehensive guide – Learn Python Programming Basics – Beginner to Master.
Python Programming – Complete Tutorial
In this Python tutorial for beginners, we have covered basic and advanced topics of Python. We have covered multiple scenarios for each topic and provided program syntax for that. We have also covered multiple programs on each topics written in Python. Lets learn all the topics covered in this Python Tutorial.
Python Installation and Development Environment
Python is a popular, multi-purpose, versatile and easy-to-learn high-level programming language with a simple and readable syntax. Python supports several key programming paradigms and was designed & built with core principals. To start programming with Python 3, we need to install Python 3 on Windows by downloading it from the official website. We can also install Python 3 on Ubuntu via simple terminal commands. Once a Python 3 is installed, we can setup our Python development environment by setting up Python IDE such as PyCharm, Visual Studio Code, or Jupyter Notebook.
Data Types in Python
Python has several built-in data types that help manage and manipulate different kinds of data:
- Number Data types
- Integer (int) – Represents whole numbers, e.g.,
5
,100
,-10
. - Float (float) – Represents decimal or floating-point numbers, e.g.,
3.14
,-0.001
.
- Integer (int) – Represents whole numbers, e.g.,
- String (str) – Represents text, enclosed in single or double quotes, e.g.,
'hello'
,"Python"
. - Boolean (bool) – Represents truth values,
True
orFalse
. - List – An ordered, mutable collection of items, e.g.,
[1, 2, 3]
. - Tuple – An ordered, immutable collection, e.g.,
(1, 2, 3)
. - Set – An unordered, unique collection of elements, e.g.,
{1, 2, 3}
. - Dictionary (dict) – A collection of key-value pairs, e.g.,
{'name': 'shbytes', 'tutorials': ["Python"]}
. - Array – Python programming provides the
array
module, which are very similar to List.
These data types enable Python to handle a variety of tasks efficiently, from simple calculations to complex data processing. Understanding of variables scopes is also very important to work efficiently with Python.

Understanding Lists in Python
List is a core datatype in Python. List are sequences which are used to store elements (collection of data). Lists are similar to Arrays but are of dynamic size. It means size of list can be increase or decreased as we add or remove elements from the list.
List is a core datatypes in Python along with Tuple, Set and Dictionary. Lists in Python are of <class ‘list’>. Lists in Python are mutable objects, it means we can perform add (append), update (change), delete (remove) operations on the lists objects.
As part of this Learn Python Programming Basics tutorial, we have covered different operations using programs that we can perform on Lists in Python.
Tuples in Python: Operations, Definition & Examples
Tuple are sequences which are used to store elements (collection of data). Similar to Arrays and Lists, Tuples also store elements based on index and can be access based on the index position.
Tuple is a core datatypes in Python along with List, Set and Dictionary. Tuples in Python are of <class ‘tuple’>. Tuple in Python are immutable objects. It means we cannot perform add (append), update (change), delete (remove) operations on the tuple objects. Size of tuple objects cannot be increase or decreased as we cannot add or remove elements from the tuple.
As part of this Learn Python Programming Basics tutorial, we have covered different operations using programs that we can perform on Tuples in Python.
Set in Python
- Set is an unordered collection datatype. Unlike List and Tuple, elements in Set are not stored based on their index positions.
- Sets can store heterogeneous elements i.e sets can store elements of different datatypes including the collection elements except List. Set does not allow storing List as an element.
- Set is iterable collection. We can iterate through the elements in Set. But, there is no order defined for elements in Set. During iteration elements can come in any order.
- Set is mutable collection datatype. It means we can perform add (append), update (change), delete (remove) operations on the set objects.
- Set does not allow duplicate elements. Sets will always store unique elements. But we can store different case-sensitive elements in it. Elimination of duplicate elements from a collection is the primary use case of Set.
- Set in Python support mathematical operations like union, intersection, difference, and symmetric difference, subset and super-set.
As part of this Learn Python Programming Basics tutorial, we have covered different operations using programs that we can perform on Sets in Python.
Dictionary in Python
- Key-Value – Dictionary store data in key-value pairs. We can store multiple
key-value
pair elements, of different datatypes. - Unordered – Similar to Set, Dictionary is Python is also an unordered collection datatype. Dictionary elements (key-value) have no fixed order and do not maintain the sequence of its elements. From Python 3.8, dictionaries allowed to maintain the insertion order of its elements.
- Mutable – Dictionary is a mutable collection datatype. It means we can perform add (append), update (change), delete (remove) operations on the dictionary objects.
- Indexed by keys: Dictionary elements are indexed by keys. As a key-pair element, keys are indexed and values are stored for those keys (index). We can use keys to access its corresponding value.
- Immutable keys – Dictionary element keys must be unique and of immutable types (e.g., strings, numbers, and tuples). Key values can be of any datatype. Mutable datatypes like lists, sets or dictionaries cannot be used as keys.
- Dynamic size – Dictionary in Python are mutable datatype. We can perform add, update or remove operations on dictionary. Size of dictionary (number of elements in dictionary) can increase or decrease based on these operations.
- Fast lookup – Dictionary elements are key-value pair and indexed based on keys. This feature helps to provide fast searching, insertion and deletion operation within the dictionary. Because of key based indexing (hashing technique) these operations can be performed in O(1) time complexity.
- Keys, values and items iteration – Dictionary allows to traverse over all the elements. We can iterate over keys, value and items (key-value) pair of dictionaries.
- Nested Dictionary – Dictionary allows another dictionary to be stored as a value. Another dictionary cannot be used as a key.
- From Python version 3.7 and later, dictionaries maintain insertion order.
As part of this Learn Python Programming Basics tutorial, we have covered different operations using programs that we can perform on Dictionary in Python.
Array in Python
Array is a most fundamental and useful data structure in Python Programming Language. Python programming provides the array
module, which allows to create arrays.
- Homogeneous Data – Python arrays support only single datatype elements. All elements stored in an array, should be of specific datatype (e.g., integers, floating-point numbers, characters).
- Type Codes – Array elements datatype is specified by the Type Codes. For example,
'i'
for integers and'f'
for floating-point numbers. - Memory Optimized Storage – Arrays in Python are more memory-efficient than lists. This is very helpful in handling of large amounts of data. Because of uniform datatype elements in array, Python can allocate memory more efficiently, reducing overhead.
- Contiguous Memory Layout – Array elements are stored sequentially in memory. This helps to improve data cache and performance of applications.
- Fixed Size – Specific size (number of elements) is defined when an array object is created and memory allocation is also based on the defined number of elements.
- Dynamic Resize – Append or insert operations are allowed on array object, but this operation will involve reallocation of memory. This dynamic resize reduces performance of arrays and make them less efficient than lists.
- Indexing – Arrays are indexed and its elements can be accessed based on their index positions. Arrays can achieve O(1) time complexity for accessing elements by index.
- Basic Operations: Python arrays support a range of operations similar to lists, such as slicing, concatenation, and iteration except some of the advanced functionalities provided by lists.
- Immutable Type Code – Arrays type code is immutable and cannot be changed. It means, once an array is created with a specific type code, we cannot change the type code for this array. We can delete this array and can create a new array with different type code.
- 1D Arrays – By default, Python built-in array module supports only one-dimensional arrays. For multidimensional arrays support we can use Python library NumPy.
- Interoperability with C – Python arrays can easily interacting with C libraries can be very helpful to pass data to and from C code.
As part of this Learn Python Programming Basics tutorial, we have covered different operations using programs that we can perform on Array in Python.
Collections in Python
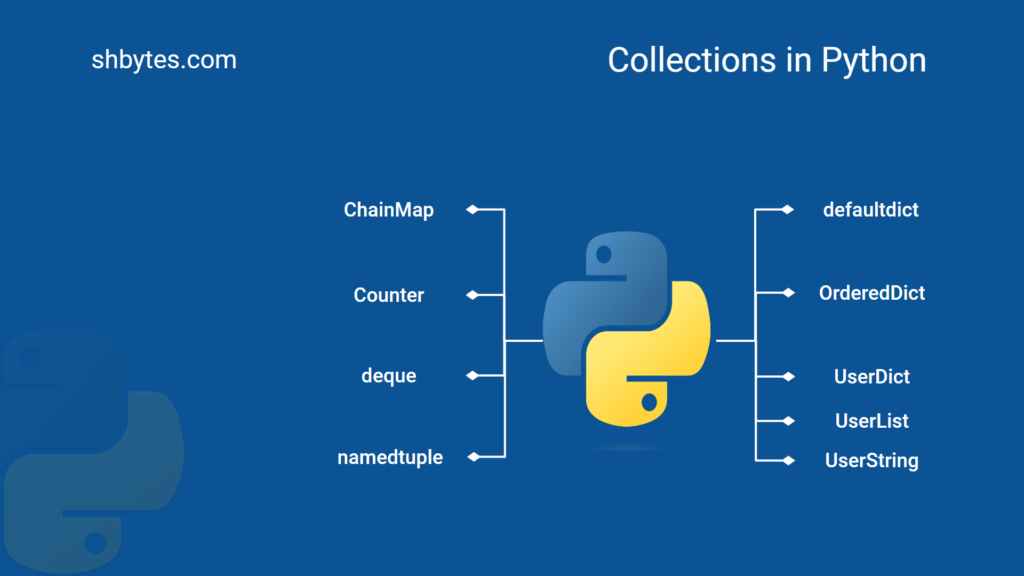
In Python, the collections
module provides alternatives to Python’s built-in container types like dict
, list
, and tuple
. These alternatives are often more flexible, efficient, or feature-rich than their basic counterparts. Some of the main classes and types provided by the collections
module, along with their key properties. We have covered collections
module extensively as part of this Python tutorial for beginners.
Hi,
I’m glad to hear from you i find great job
Best wishes,
Lay
Interesting