List slicing in Python or slicing of list in Python means extracting a part (or slice) of the list. Slicing of list uses the index position of elements in the list. Slicing can be done using either of two methods:
- Using the colon (
:
) operator - Using the
slice()
method
In previous articles, we learned about accessing list elements by index, using the list index method to find the index of a given element, appending elements to lists and updating list elements.
In this article, we will explore list slicing in Python by using index position of elements in the list.
Methods for List Slicing in Python
Slicing using the Colon (:
) Operator – We can use Python slice operator => colon (:
) along with list elements index position. Syntax for slicing using the colon (:
) operator
list[start:end:step]
Slicing using the slice()
Method – We can use Python list slice()
method along with list elements index position. Syntax for slicing using the slice()
method
list[slice(start, end, step)]
start
(optional) – This is an optional parameter that determines the starting index for slicing.
- If
start
indices is not provided, then slicing will start from the first element (i.e. index0
) of the list. - The
start
indices can be defined using positive or negative indexing - If
start
indices is not given, then index0
is taken asstart
index.
end
– This is required with slice()
method. But is optional with colon (:
) operator. The end
index slices the list up-to this index position.
- If
end
indices is not given, then slicing continues to the last element of the list. - The slicing includes elements up-to
[end - 1]
index. end
indices position can be defined using positive or negative indexing.- The default value of
end
indices is the total number of elements in the list.
step
(optional) – The step
parameter defines the interval between each index for selecting the next element in the sliced list. The default value for step
is 1.
Return value – The slicing operation returns a new list containing the sliced elements. The original list remains unchanged. If we don’t have any element in sliced list (i.e. the slice list is empty), then no error is raised.
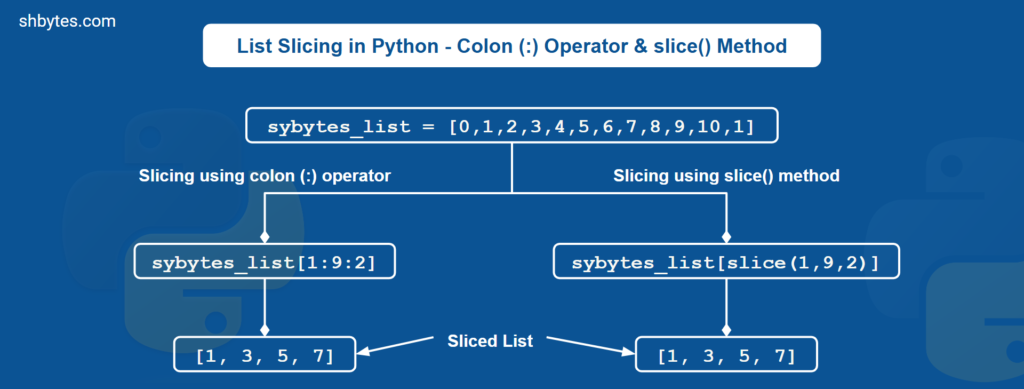
Program – List Slicing in Python
# slicing range will be from index 1 to 8 (end - 1) and step of 2
sybytes_list=[0,1,2,3,4,5,6,7,8,9,10,1]
print(sybytes_list[1:9:2]) # colon operator, start index 1, end index 9, step by 2
print(sybytes_list[slice(1, 9, 2)]) # slice method, start index 1, end index 9, step by 2
print(sybytes_list)
Output – Example Program – List Slicing in Python
[1, 3, 5, 7] # sliced list using colon operator
[1, 3, 5, 7] # sliced list using slice() method
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 1]
Slicing of List – No Error Raised
Slicing of list will not raise an error, even if the given end
indices is out of range from the list.
# slicing will not raise an error, if end indices is greater than the number of elements in the list
sybytes_list = [0,1,2,3,4,5,6,7,8,9,10,1]
print("slicing will not throw an error, if end index is out of range from the list")
print(sybytes_list[2:14])
print(sybytes_list[slice(2, 14)])
print(sybytes_list)
In this example program, the defined sybytes_list
has a total of 12 elements, and we are performing slicing on that list. The end
indices provided is 14
, which is out of range for the sybytes_list
. However, slicing will not raise an error and will slice the list up to the last element. By default, the step
indices value will be set to 1
.
Output – Example Program – Slicing of List – No Error Raised
slicing will not throw an error, if end index is out of range from the list
[2, 3, 4, 5, 6, 7, 8, 9, 10, 1] # sliced list using colon operator
[2, 3, 4, 5, 6, 7, 8, 9, 10, 1] # sliced list using slice() method
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 1]
From the output, all the elements from index 2
to the last index in list are taken in sliced list. No error is raised even if the given end
indices was out of range from list.
Program – Slicing to End of the List
- With the Python slice operator – colon (
:
), we don’t need to provideend
indices. By defaultend
index is number of elements in the list. - With the
slice()
method, we can provide end index either with positive index or a negative index. However, using a negative index will exclude the last element of the list, because slicing in Python works from the start to (end – 1) index only.
# Slicing to End of the List
sybytes_list = [0,1,2,3,4,5,6,7,8,9,10,1]
print("slicing to end of list")
print(sybytes_list[1:]) # colon operator, end index not required
print(sybytes_list[slice(1, -1)]) # slice method, end index with negative indexing
print(sybytes_list[slice(1, 12)]) # slice method, end index with positive indexing
print(sybytes_list)
The defined sybytes_list
contains a total of 12 elements. With the colon :
operator, we specify the start index and leave the end index optional. With the slice()
method, we use -1
(negative indexing) or 12
(the total number of elements in the list) for the end index.
Output – Example Program – Slicing to End of the List
slicing to end of list
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 1]
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10] # sliced list using colon operator, end index not required
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 1] # sliced list using slice() method - end index with negative indexing
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 1] # sliced list using slice() method - end index with positive indexing
As an output, We got all elements starting from index 2
. But in case of slice()
method with negative indexing we miss out on the last element. Elements are taken from start to end -1
index only.
Program – Slicing Till Given Index of List
To slice till given index:
- With the colon
:
operator orslice()
method, in both ways there is no need to provide thestart
indices. By default, thestart
index is0
. - With the
slice()
method, we can specify only the end index, which can be provided either with a positive or negative index.
# Slicing Till Given Index of List
sybytes_list = [0,1,2,3,4,5,6,7,8,9,10,1]
print("slicing value from index 0 to 3 of the list")
print(sybytes_list[:4]) # colon operator, start index not required
print(sybytes_list[slice(4)]) # slice method, start index not required
print(sybytes_list, type(sybytes_list))
With colon operator, we provide end index prefix with colon and no need to provide start index. With ‘slice’ method we provided end index only. When only one parameter is given with ‘slice’ method it is taken for end index.
- With the colon operator, we provide the
end
indices prefixed with a colon and do not need to provide thestart
index. - With the
slice()
method, we provide only theend
indices. When only one parameter is given with theslice()
method, it is treated as theend
index.
Output – Example Program – Slicing Till Given Index of List
slicing value from index 0 to 3 of the list
[0, 1, 2, 3] # slicing with colon operator - Positive End Indices in Slicing
[0, 1, 2, 3] # slicing with slice() method - Positive End Indices in Slicing
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 1] <class 'list'>
From the output, with colon operator and slice()
method both, we got elements starting from index 0
till end index 3 [4 - 1]
.
Negative End Indices in Slicing
You can use negative indices to slice up to a certain element from the end
.
# Negative End Indices in Slicing
print("Slicing end index - Negative indexing")
sybytes_list = [0,1,2,3,4,5,6,7,8,9,10,1]
print(sybytes_list, type(sybytes_list))
print(sybytes_list[:-1]) # colon operator, end index with negative indexing
print(sybytes_list[slice(-1)]) # slice method, end index with negative indexing
With colon operator, we provide end index prefix with colon and no need to provide start index. End index is provided with negative indexing. With ‘slice’ method we provided end index only. When only one parameter is given with ‘slice’ method it is taken for end index.
- With the colon operator, we provide the
end
index prefixed with a colon and do not need to provide thestart
index. Theend
index can be provided using negative indexing. - With the
slice()
method, we provide only theend
index. When only one parameter is given with theslice()
method, it is taken as theend
index.
Output – Example Program – Negative End Indices in Slicing
Slicing end index - Negative indexing
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 1] <class 'list'>
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10] # slicing with colon operator - Negative End Indices in Slicing
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10] # slicing with slice() method - Negative End Indices in Slicing
NOTE – Even when we used negative indexing, elements are given from left to right.
Negative Indices in Slicing
# Negative Indices in Slicing
print("Slicing start and end index - Negative indexing")
sybytes_list = [0,1,2,3,4,5,6,7,8,9,10,1]
print(sybytes_list, type(sybytes_list))
print(sybytes_list[-3:-1]) # colon operator, start & end index, negative indexing
print(sybytes_list[slice(-3,-1)]) # slice method, start & end index, negative indexing
Output – Example Program – Negative Indices in Slicing
Slicing start and end index - Negative indexing
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 1] <class 'list'>
[9, 10] # Slicing with colon operator using - Negative Start and End Indices
[9, 10] # Slicing with slice() method using - Negative Start and End Indices
NOTE – Even when we used negative indexing, elements are given from left to right.
Slicing with Step – Positive Step (Left to Right)
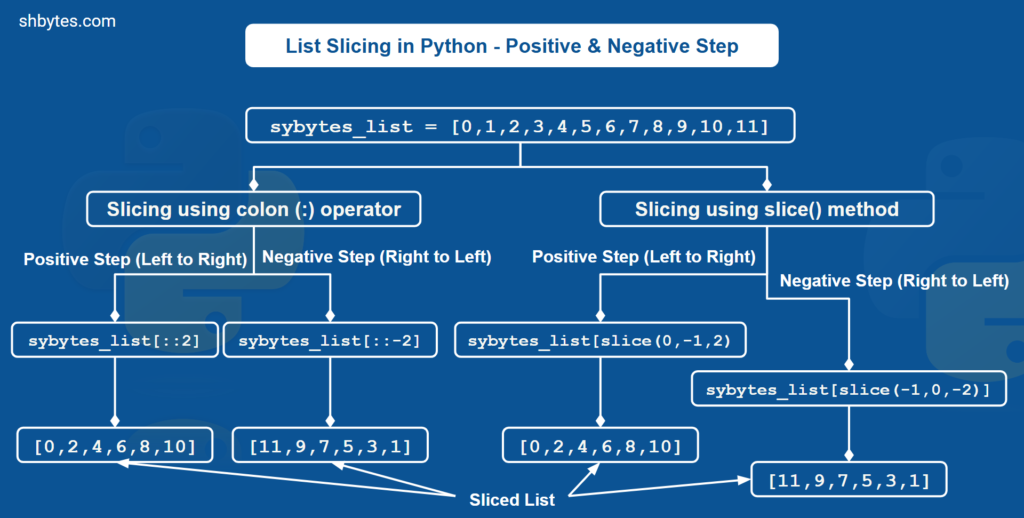
You can specify a positive step
to slice elements in a positive direction (left to right).
# Slicing with a Positive Step (Left to Right)
print("Slicing left to right - Positive step")
sybytes_list = [0,1,2,3,4,5,6,7,8,9,10,11]
print(sybytes_list, type(sybytes_list))
print(sybytes_list[::2]) # colon operator, positive step, left to right
print(sybytes_list[slice(0, -1, 2)]) # slice method, positive step, left to right
- Slicing with the colon operator, we are not using
start
andend
indices but only thestep
value of2
. Note – empty colon for start and end. - Slicing with the
slice()
method, we need to provide thestart
andend
indices along with thestep
value of2
. step
value2
means, slicing will jump 2 steps (from left to right) to select the next element.
Output – Example Program – Slicing with a Positive Step (Left to Right)
Slicing left to right - Positive step
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11] <class 'list'>
[0, 2, 4, 6, 8, 10] # Positive step (left to right) - sliced list using colon operator
[0, 2, 4, 6, 8, 10] # Positive step (left to right) - sliced list using slice() method
With step
2
, slicing is jumping 2 steps (from left to right) to select the next element. From the output, we can see alternate elements in the sliced list. Because of the positive step
, elements are selected in left to right direction.
Slicing with Step – Negative Step (Right to Left)
- Negative
steps
allow you to slice the list in reverse order i.e from right to left.
# Slicing with a Negative Step (Right to Left)
print("Slicing right to left - Negative step")
sybytes_list=[0,1,2,3,4,5,6,7,8,9,10,11]
print(sybytes_list, type(sybytes_list))
print(sybytes_list[::-2]) # colon operator, negative step, right to left
print(sybytes_list[slice(-1, 0, -2)]) # slice() method, negative step, right to left
step
value-2
means, slicing will jump 2 steps in right to left direction to select the next element.
Output – Example Program – Slicing with a Negative Step (Right to Left)
Slicing right to left - Negative step
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11] <class 'list'>
[11, 9, 7, 5, 3, 1] # Negative step (right to left) - sliced list using colon operator
[11, 9, 7, 5, 3, 1] # Negative step (right to left) - sliced list using slice() method
With step
-2
, slicing is taking 2 steps in the right to left direction to select the next element. From the output, we can see alternate elements in the sliced list. Because of negative step, elements are taken in the right to left direction.
Summary
In this article, we explored various methods of slicing a list in Python, including:
- Slicing using the colon operator and slice() method
- Handling out-of-range indices gracefully
- Slicing to the end or till a specific index
- Using negative indices and steps for more complex slicing
Code snippets and programs related to List Slicing in Python, can be accessed from GitHub Repository. This GitHub repository all contains programs related to other topics in Python tutorial.
Interview Questions & Answers
Q: How to modify a list using slicing?
Use slicing to modify parts of a list by assigning new values to a slice. The length of the slice on the left-hand side can be different from the length of the values on the right-hand side. Following example shows two scenarios:
- Using slicing – Replace multiple elements of list with multiple new elements from another list
- Using slicing – Replace multiple elements of list with a single element
number_list = [12, 24, 36, 48, 58]
number_list[1:4] = [21, 31, 41] # Replace elements from index 1 to 3
print(number_list) # number_list after replacing elements: [10, 21, 31, 41, 50]
number_list[1:3] = [22] # Replace elements at index 1 and 2 with a single element
print(number_list) # number_list after replacing elements: [10, 22, 41, 50]
Q: How to use slicing to insert elements into a list?
We can insert elements into a list by assigning values to an empty slice. Empty slice can be defined which has the same start and end index position. Slice should have a start and end index set to the position where we want to insert the elements.
number_list = [12, 24, 36, 48, 58]
number_list[2:2] = [25, 26] # Insert [25, 26] at index 2
print(number_list) # number_list elements after inserting: [12, 24, 25, 26, 36, 48, 58]
Note – In this example, number_list[2:2]
has same start and end index position.
Q: How can we delete elements from a list using slicing?
There are two ways, we can delete elements from a list using slicing.
- By assigning an empty list (
[]
) to the slice to be deleted
number_list = [12, 24, 36, 48, 58]
number_list[1:3] = []
print(number_list) # number_list elements after deleting: [12, 48, 58]
- By using the
del
statement for the slice to be deleted
number_list = [12, 24, 36, 48, 58]
del number_list[1:3]
print(number_list) # number_list elements after deleting: [12, 48, 58]
Q: Is it possible to use slicing with a list of objects or custom classes?
Yes, list slicing in Python is not limited to lists of primitive types like integers or strings. We can slice lists containing objects of custom classes or any other data types.
class ShbytesClass:
def __init__(self, value):
self.value = value
def __repr__(self):
return f"ShbytesClass({self.value})"
obj_list = [ShbytesClass(1), ShbytesClass(2), ShbytesClass(3), ShbytesClass(4), ShbytesClass(5)]
slice = obj_list[1:4]
print(slice) # Output: [ShbytesClass(2), ShbytesClass(3), ShbytesClass(4)]
Q: Explain how slicing works with multidimensional lists (i.e., lists of lists).
When working with multidimensional lists (lists of lists), slicing will operate on the outermost list by default. To slice inner lists, we need to first get the inner list and then apply slicing on it.
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9],
[10, 11, 12]
]
# Slicing the outer list
outer_slice = matrix[1:3]
print(outer_slice) # slice elements from outer list: [[4, 5, 6], [7, 8, 9]]
# Slicing an inner list
inner_slice = matrix[1][1:3]
print(inner_slice) # slice elements from inner list: [5, 6]
In the example, first slice matrix[1:3]
returns slice index from outer list. The second slice matrix[1][1:3]
take out the inner list first and then slice index from that list.
Test Your Knowledge: Practice Quiz
Related Topics
- Understanding Lists in Python: A Comprehensive GuideLists in Python List is a core datatype in Python. Lists are sequences used to store elements (collection of data). Lists are similar to Arrays but are of dynamic size. It means the size of the list can be increased or decreased as we add or remove elements from the list. Understanding Python Lists: A…
- How to Create List in Python | Python List Creation Methods ExplainedList is a sequence data type used to store elements (collection of data). Lists are similar to Arrays but have a dynamic size, meaning the list size can increase or decrease as elements are added or removed. A list is a core data type in Python, along with Tuple, Set and Dictionary. Lists in Python…
- How to Access List Elements by Index in Python (with Example Programs)Python list elements are stored based on their index positions and can be accessed by their index positions, which allows to quickly retrieve specific element from the list. Index assignment to list element in Python, is similar to index assigned in Array elements. Understanding how to access list elements by index is crucial for data…
- Python List Index Method Explained with Examples | Get Element Position in ListElements in a Python list are stored based on their index positions. Lists in Python provides an index(arg) method to get the index position of an element within a list. In the previous article How to Access List Elements by Index, we learned how to access a list element using its index position. In this…
- Append Elements to List – Python | Add Elements with Append MethodIn Python, elements in a list can be added using the append(element) method. This method allows us to add elements to the end of a list easily. In the previous articles, we explored accessing list elements by index and using the list index method to retrieve an element’s position. This article covers appending new elements…