Subset and Superset are two fundamental concepts in set theory. Python also has built-in methods to support these concepts.
Subsets
A set is defined as the subset of another set, if first set all elements are also elements of second set. i.e. set A
will be the subset of set B
if all elements of set A
are also elements of set B
.
Subset – Mathematical Definition
Subset is denoted with symbol ⊆
. Above definition can be denoted as A ⊆ B
. If set A
is a subset of set B
, then it means that set A
and set B
are either equal OR all elements of set A
are also elements of set B
.
In Mathematics, subset can be defined as A ⊆ B ⟺ ∀ x ( x ∈ A ⟹ x ∈ B )
. Lets understand this with an example:
A = {10, 20} and B = {10, 20, 30}
Here, A ⊆ B
because both elements of set A (10 and 20) are also elements of set B.
- The empty set
∅
is a subset of all the sets, including itself. For any set B,∅ ⊆ B
.
Set A subset of Set B
=> A ⊆ B
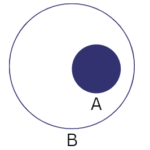
Subset symbol ⊆
also includes equality in it. This leads to the concept of Proper Subset.
Proper Subset: set A
is a proper subset of set B
, if A ⊆ B
and A ≠ B
. This is denoted as A ⊂ B
. Example – A = {10, 20}
and B = {10, 20, 30}
. Here, A ⊂ B
because set A is contained within set B and A ≠ B
.
Properties of Subsets
- Reflexivity: Every set is always a subset of itself,
A ⊆ A
. - Transitivity: If
A ⊆ B
andB ⊆ C
, thenA ⊆ C
. It means, if set A is a subset of set B and set B is a subset of set C, then it make set A subset of Set C also. Example –A = {10, 20}, B = {10, 20, 30}, C = {10, 20, 30, 40}
- Antisymmetry: If
A ⊆ B
andB ⊆ A
, thenA = B
. It means, if set A is a subset of set B and set B is a subset of set A, then both set A and set B should be equal. Example –A = {10, 20}, B = {10, 20}
Python provides built-in method issubset()
to check if the set is subset to another set or not. Lets see, how issubset()
method works in Python.
issubset()
method
Using - Syntax –
set_1.issubset(set_2)
issubset()
method takes a single “iterable” as an argument.set_1.issubset(set_2)
method returns boolean (True
orFalse
) value.True
meansset_1
is a subset ofset_2
andFalse
meansset_1
is not a subset ofset_2
.
# using issubset() method
print("using issubset() method")
set_1 = {10, 20, 30}
set_2 = {10, 20, 30, 70, 80, 90}
subset_set12 = set_1.issubset(set_2) # check set_1 is subset of set_2
subset_set21 = set_2.issubset(set_1) # check set_2 is subset of set_1
print(set_1)
print(set_2)
print(subset_set12) # return value for set_1 is subset of set_2
print(subset_set21) # return value for set_2 is subset of set_1
We have defined two sets referenced by variables set_1
and set_2
. Using built-in issubset()
method we are checking if set_1
and set_2
are subset to each other or not. Output from issubset()
method will be a boolean value.
Program Output
using issubset() method
{10, 20, 30} # elements of set_1
{80, 20, 90, 70, 10, 30} # elements of set_2
True # set_1 is subset of set_2
False # set_2 is subset of set_1
From the program output, set_1
and set_2
elements are printed. issubset()
method has return a boolean (True
or False
) value. True
for set_1
is subset of set_2
and False
when set_2
is not subset of set_1
.
issubset()
method – with empty sets
Using - Syntax –
set_1.issubset(set_2)
# using issubset() method - with empty set
print("using issubset() method - with empty set")
set_1 = set()
set_2 = set()
subset_set12 = set_1.issubset(set_2) # check set_1 is subset of set_2
subset_set21 = set_2.issubset(set_1) # check set_2 is subset of set_1
print(set_1)
print(set_2)
print(subset_set12) # will return True = set_1 is subset of set_2
print(subset_set21) # will return True = set_2 is subset of set_1
We have defined two sets referenced by variables set_1
and set_2
. Both sets are empty sets. Using built-in issubset()
method we are checking if set_1
is subset of set_2
OR set_2
is subset of set_1
. Output from issubset()
method will be a boolean value.
Program Output
using issubset() method - with empty set
set() # elements of set_1
set() # elements of set_2
True # set_1 is subset of set_2
True # set_2 is subset of set_1
Since both set_1
and set_2
are empty sets, so they are subset to each other. As an output we got True for both set_1
subset of set_2
and set_2
subset of set_1
.
<=
) operator
Using subset (- Syntax –
set_1 <= set_2
set_1 <= set_2
returns boolean (True
orFalse
) value.True
meansset_1
is a subset ofset_2
andFalse
meansset_1
is not a subset ofset_2
.
# using subset (<=) operator
print("using subset (<=) operator")
set_1 = {10, 20, 30}
set_2 = {10, 20, 30, 70, 80, 90}
subset_set12 = set_1 <= set_2 # check set_1 is subset of set_2
subset_set21 = set_2 <= set_1 # check set_2 is subset of set_1
print(set_1)
print(set_2)
print(subset_set12) # will return True = set_1 is subset of set_2
print(subset_set21) # will return False = set_2 is not subset of set_1
We have defined two sets referenced by variables set_1
and set_2
. Using subset (<=
) operator, we are checking if set_1
and set_2
are subset to each other or not. Output from subset (<=
) operator will be a boolean value.
Program Output
using subset (<=) operator
{10, 20, 30} # elements of set_1
{80, 20, 90, 70, 10, 30} # elements of set_2
True # set_1 is subset of set_2
False # set_2 is subset of set_1
From the program output, set_1
and set_2
elements are printed. subset (<=
) operator has return a boolean (True
or False
) value. True
for set_1
is subset of set_2
and False
for set_2
is subset of set_1
.
<
) operator
Using proper (strict) subset (- Syntax –
set_1 < set_2
(Note – we have only less than operator, equal to sign is not with it) set_1 < set_2
returns boolean (True
orFalse
) value.True
meansset_1
is a subset ofset_2
andFalse
meansset_1
is not a subset ofset_2
.- This operator is useful to check if set_1 is proper subset of set_2 or not.
# using strict subset (<) operator
print("using strict subset (<=) operator")
set_1 = {10, 20, 30}
set_2 = {10, 20, 30, 70, 80, 90}
subset_set12 = set_1 < set_2 # check set_1 is proper subset of set_2
subset_set21 = set_2 < set_1 # check set_2 is proper subset of set_1
print(set_1)
print(set_2)
print(subset_set12) # will return True = set_1 is subset of set_2
print(subset_set21) # will return False = set_2 is not subset of set_1
We have defined two sets referenced by variables set_1
and set_2
. Using proper (strict) subset (<
) operator, we are checking if set_1
and set_2
are proper subset to each other or not. Output from proper (strict) subset (<
) operator will be a boolean value.
Program Output
using strict subset (<=) operator
{10, 20, 30} # elements of set_1
{80, 20, 90, 70, 10, 30} # elements of set_2
True # set_1 is subset of set_2
False # set_2 is subset of set_1
From the program output, set_1
and set_2
elements are printed. Proper (strict) subset (<
) operator has return a boolean (True
or False
) value. True
for set_1
is proper subset of set_2
and False
shows that set_2
is not proper subset of set_1
.
issubset
() method
Custom implementation – Internally in Python, issubset()
method checks for all elements of set_1
are present in set_2
. If any element of set_1
is not present in set_2
, then method returns False
; otherwise, it returns True
.
Lets see the custom code to implement functioning of issubset()
method.
def custom_issubset(set_1, set_2):
# Iterate through elements of set_1
for element in set_1:
# Check if any element of set_1 not in set_2
if element not in set_2:
return False
return True
set_1 = {10, 20, 30}
set_2 = {10, 20, 30, 40, 50, 60}
set_3 = set() # check for empty sets
set_4 = set() # check for empty sets
print(custom_issubset(set_1, set_2)) # Output: True
print(custom_issubset(set_2, set_1)) # Output: False
print(custom_issubset(set_3, set_4)) # Output: True, check for empty sets
custom_issubset()
function iterates through each element inset_1
.- For each element in
set_1
, it checks if the element is present inset_2
or not. - If any element is not found in
set_2
, the function returnsFalse
, else it will continue to check for all the elements ofset_1
. If all elements ofset_1
are present inset_2
, then it will returnTrue
.
Summary
In this article we learn about various subset operation on Sets. Following scenarios were explored:
- Subset – Mathematical Definition
- Using issubset() method
- Using issubset() method – with empty sets
- Using subset (<=) operator
- Using proper (strict) subset (<) operator
- Custom implementation – issubset() method
Code – Github Repository
All code snippets and programs for this article and for Python tutorial, can be accessed from Github repository – Comments and Docstring in Python.
Python Topics
Interview Questions & Answers
issubset()
with a non-set type, like a list or a dictionary?
Q: What happens when we use issubset()
method can be used with other iterables like lists or dictionaries.
- With lists, it checks if all elements of the set are present in the list.
- With dictionaries it checks if all elements of the set are present as the dictionary keys.
set_1 = {10, 20, 30}
list_1 = [10, 20, 30, 40]
print(set_1.issubset(list_1)) # True
dict_1 = {10: 'Python', 20: 'Power BI', 30: 'LLM'}
print(set_1.issubset(dict_1)) # True
issubset()
method?
Q: What is the time complexity of the Time complexity of issubset()
method is O(len(set_1))
where set_1
is the set being checked for subset of another set or iterable. Python iterate over all elements of set set_1
and check if each element is present in another set. Since, sets are implemented as hash sets, time complexity to check membership is O(1).
issubset()
method can be used?
Q: Provide a real-world example where Consider a scenario where you have a list of skills required for a job and a list of skills a candidate possesses. You want to check if the candidate is qualified for the job by ensuring that all required skills are present in the candidate’s skill set.
issubset()
method?
Q: What are the common mistakes when using the - Confuse with other methods – Developers might confuse
issubset()
with other set operations likeissuperset()
, which checks the superset condition, which is opposite to subset condition. - Type of iterables –
issubset()
can work with other iterables like lists, it’s important to understand how Python interprets this. For instance, usingissubset()
on a dictionary will only consider the keys, not the values. - Mutable elements in sets – Sets in Python cannot contain mutable elements like lists. Using
issubset()
with a set containing such elements will raise an error.