What are Variables in Python
In Python, variables act as references to reserved memory locations where actual values are stored. Each variable name points to a specific memory address that holds the assigned value.
In this case:
height
is a variable name referencing a memory location where 175 (its value) is stored.- The memory address might look like 0x0041F2 (example for illustration).
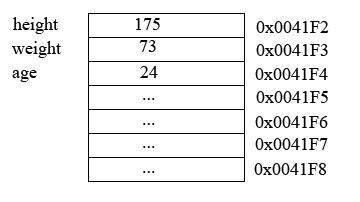
How Variable Memory Works
When we define variables, like height = 175, Python allocates a specific memory location to store value 175 and assigns the variable height
as a reference to this location. Here, height acts as a pointer to the memory address containing the value 175.
For other variables, such as – weight = 73 refers to a separate memory address, such as 0x0041F3 (illustrative), storing the value 73.
In Python, this system of referencing memory locations by variable names allows dynamic handling of values and memory, which is essential for Python’s flexibility.
Declaration and Initialization of Variables in Python
- Declaration of a variable
The declaration of a variable refers to allocating a memory location without assigning a value. This memory location will act as a reference (or pointer) for the defined variable. At this stage, the allocated memory does not store any specific value.
- Initialization of a variable
The initialization of a variable involves storing a value in the memory location referenced (or pointed to) by the defined variable.
In Python, variables can be declared and initialized simultaneously, meaning that variables are declared as soon as a value is assigned to them.
# Declaration and Initialization of variables
# This will initialize variable x with value of 10
x = 10
print(x)
# This will re-declare the variable x
x = "Hello Shbytes"
print(x)
Dynamically Typed Variables in Python vs Java
Python
Java
- Python is a dynamically typed language, meaning:
- Variables can be declared and initialized without specifying a data type.
- The data type is determined based on the variable’s assigned value, allowing flexibility in type.
- In Python, variables do not have a fixed data type and can be re-declared with values of different data types.
- Java, on the other hand, is a statically typed language, meaning:
- Variables must be declared with a specific data type.
- Variables can only reference values of their defined data type and cannot be reassigned with a different data type value.
This difference allows Python greater flexibility in variable usage, while Java enforces stricter data type constraints.
Code reference
# Declaration and Initialization of variables
# This will initialize variable x with value of 10
x = 10
print(x)
print(type(x))
print("\n---------------------------------------\n")
# This will re-declare the variable x
x = "Shbytes"
print(x)
print(type(x))
Here, datatype of variable x is not defined, but it refers to a memory address of value 10, which is an integer. Datatype of variable x is int (shorthand for integer).
In second case, we change the value of x to a string, x = “Shbytes”. Datatype of variable x also changed to str (shorthand for string).
Following the output for the above code block:
10
<class 'int'>
---------------------------------------
Shbytes
<class 'str'>
Variable Data Types in Python
Now, we will explore the core data types for variables in Python. The Python programming language provides several core data types for variables:
- Number
- Boolean
- String
- List
- Tuple
- Set
- Dictionary
Custom types can be created by using the existing base classes.
Program to show Data Types in Python
This program demonstrates Python variables initialized with different data types and displays their data type as defined by Python. Each variable’s data type is identified by its Python class:
- Integer – <class ‘int’>
- String – <class ‘str’>
- List – <class ‘list’>
- Dictionary – <class ‘dict’>
- Set – <class ‘set’>
- Tuple – <class ‘tuple’>
- Boolean – <class ‘bool’>
m = 30 # initialize variable m
print(m) # display m:
print(type(m)) # display the data type of m:
print("\n---------------------------------------\n")
m = "Hello Shbytes"
print(m)
print(type(m)) # variable data type <class 'str'>
print("\n---------------------------------------\n")
m = ["Python", "Java", ".net"]
print(m)
print(type(m)) # variable data type <class 'list'>
print("\n---------------------------------------\n")
m = {"course" : "Python", "delevered_by" : "Shbytes"}
print(m)
print(type(m)) # variable data type <class 'dict'>
print("\n---------------------------------------\n")
m = {"session1", "session2", "session3"}
print(m)
print(type(m)) # variable data type <class 'set'>
print("\n---------------------------------------\n")
m = ("Python", "java", ".net")
print(m)
print(type(m)) # variable data type <class 'tuple'>
print("\n---------------------------------------\n")
m = True
print(m)
print(type(m)) # variable data type <class 'bool'>
Output of the above program
30
<class 'int'>
---------------------------------------
Hello Shbytes
<class 'str'>
---------------------------------------
['Python', 'Java', '.net']
<class 'list'>
---------------------------------------
{'course': 'Python', 'delevered_by': 'Shbytes'}
<class 'dict'>
---------------------------------------
{'session1', 'session2', 'session3'}
<class 'set'>
---------------------------------------
('Python', 'java', '.net')
<class 'tuple'>
--------------------------------------
True
<class 'bool'>
Delete Variable in Python
In Python, a variable acts as a reference (or pointer) to a memory location where its value is stored.
Using the del keyword with a variable deletes the variable reference. Once deleted, the link to the object in memory is broken, and the object is no longer referenced by any variable.
Python includes a garbage collection system (runs a daemon program) that automatically frees memory by removing un-referenced objects. After deleting a variable reference, the variable cannot be used to access the value or perform any operations.
Attempting to use a deleted variable will result in a NameError
indicating that the variable is no longer defined.
Program to Delete Variable in Python
# Variable is declared and initialized
s = "This is an object referenced by variable s"
print(s) # Print the value of the variable
del s # Delete the defined variable (Breaks the reference to the memory)
print(s) # Gives error, since variable s is already deleted
Output of the Delete variable program
This is an object referenced by variable s
Traceback (most recent call last):
File "D:\delete_variable.py", line 8, in <module>
print(s) # Gives error, since variable s is already deleted
^
NameError: name 's' is not defined
Summary
This article explains that variables in Python are references to memory locations where values are stored, allowing for dynamic declaration and initialization. Python is dynamically typed, enabling flexibility in variable types compared to Java’s static typing. It outlines core data types in Python, including Number, Boolean, String, List, Tuple, Set, and Dictionary, and includes a program to demonstrate their initialization and class identification. Finally, it describes how to delete variable in python using the del
keyword, which breaks the link to their memory locations and triggers garbage collection, resulting in a NameError
if accessed afterward.
Read article – What Are Comments and Docstring in Python (With Programs), to learn more about
- Syntax for Single line and Multi-line comments
- Declaration and access of Docstring for functions, modules and classes
- Access of Docstring using the __doc__ method on the object
- Access of Docstring using the help function passing object as an argument
Code snippets and programs related to Variables in Python and Data types in Python, can be accessed from GitHub Repository. This GitHub repository all contains programs related to other topics in Python tutorial.
Interview Questions & Answers
Q: What is the difference between mutable and immutable data types in Python?
- Mutable datatypes: Values can be changed after they are created. Lists (
list
), Dictionaries (dict
), Sets (set
). - Immutable datatypes: Values cannot be changed or altered once they are created. Strings (
str
), Tuples (tuple
), Integers (int
), Floats (float
), Boolean (bool
).
Q: How does Python handle type conversion?
In Python, Type conversion is handled in two ways:
- Implicit Conversion – Python automatically converts one datatype to another when it is necessary.
x = 30 # <class 'int'>
y = 12.5 # <class 'float'>
result = x + y # x is implicitly converted to float
print(result) # Output: 42.5
- Explicit Conversion – Manual conversion of a variable from one datatype to another using functions like
int()
,float()
,str()
, etc.
num = 50 # <class 'int'>
str_num = str(num) # Explicit conversion from int to str
print(type(str_num)) # Output: <class 'str'>
None
datatype in Python?
Q: What is the significance of the None
is a special constant in Python that represents the absence of a value or a null value. It is equivalent to Null
in other programming languages. It is used as a default value for variables or function arguments, or function doesn’t return anything. None
is of type NoneType
.
Q: Explain the concept of dynamic typing in Python.
Python is a dynamically typed language. It means datatype of a variable is determined at runtime. We don’t need to declare variable with predefined datatype. This allows to change the type of a variable by assigning it a different value. Some times, this flexibility can create security issues and requires careful handling to avoid type-related errors.
x = 10 # <class 'int'>
x = "Hello" # <class 'str'>
Test Your Knowledge: Practice Quiz
Related Topics
- Understanding Scope in Python Programming (with Example Programs)In previous articles, we learned about following topics: Understanding Scope in Programming Generally, in the scope of a program, a variable can be declared only once with the same name. But, it’s possible to declare variables with the same name in different scopes within the same program. Changing the value of a variable within a…
- Understanding Number Datatype in Python: Decimal, Octal and Hexadecimal NumbersUnderstanding the Number Datatype in Python: A Comprehensive Overview Number datatype in Python is a combination of different number format classes like Integer, Float, and Complex. Python supports the Decimal, Octal decimal, and Hexadecimal number systems. Program – Integer Number datatype Output of the Integer Number datatype program: Note from the output: Exploring Float Number…
- Introduction to Variables in Python & Variable Data TypesWhat are Variables in Python In Python, variables act as references to reserved memory locations where actual values are stored. Each variable name points to a specific memory address that holds the assigned value. In this case: How Variable Memory Works When we define variables, like height = 175, Python allocates a specific memory location…
- What Are Comments and Docstring in Python (With Programs)?Before we start learning about Comments and Docstring in Python and its concepts, we should setup our Python Integrated Development Environment (IDE) on our local machine. Read through the following articles to learn more about What is an IDE, Popular IDEs for Python and Python installation on your machine. Introduction to Comments in Python In…
- How to Install Python 3 on Ubuntu: A Comprehensive GuideTo start developing applications using Python 3, we need to install Python 3 on our machines. Article Python 3 Installation on Windows provides comprehensive details about installation of Python 3 on Windows 10 or Windows 11. Linux-based production environments are frequently used to host commercial applications, making it essential to understand the installation of Python…