Create Arrays with Predefined Values
NumPy library provides various functions to create arrays with predefined values. While creating an array, these NumPy functions helps to initialize the arrays with initial values.
In last tutorial, we learned about Key Features of NumPy Arrays in Python. In this article, we will learn about 4 functions to create arrays with predefined values.
- Create NumPy Array using
np.zeros()
- Create NumPy Array using
np.ones()
- Create NumPy Array using
np.empty()
- Create NumPy Array using
np.full()
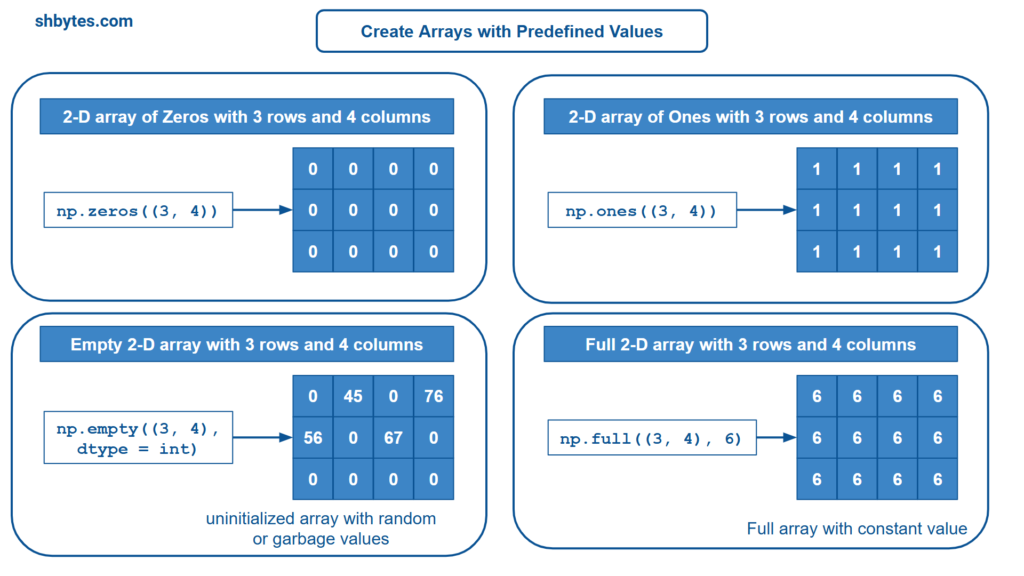
np.zeros()
Create NumPy Array using np.zeros()
creates NumPy array with all zero elements. It is mainly used to avoid uninitialized values in the array.
Syntax => np.zeros(shape, dtype=None, order='C', *args, **kwargs)
shape
(mandatory) – This is the shape or dimensions of an array to be created. An array can be created using 1-D, 2-D, 3-D or multi-dimensional array.dtype
(optional, default – float64) – The data type of an array can be int, float, or complex.order
(optional, default value – C) – The memory layout of the array can be C-style (row-major order) or F (Fortran-style (Column-major order).
import numpy as np
arr_1d_zeros = np.zeros((3,)) # 1-D array of zeros with 3 elements
print("arr_1d_zeros", arr_1d_zeros)
arr_2d_zeros = np.zeros((3, 4)) # 2-D array of zeros with 3 rows and 4 columns
print("arr_2d_zeros", arr_2d_zeros)
arr_3d_zeros = np.zeros((3, 4, 2)) # 3-D array of zeros with 3 rows, 4 columns, 2 layers
print("arr_3d_zeros", arr_3d_zeros)
# Output
# arr_1d_zeros [0. 0. 0.]
# arr_2d_zeros [[0. 0. 0. 0.][0. 0. 0. 0.][0. 0. 0. 0.]]
# arr_3d_zeros [[[0. 0.][0. 0.][0. 0.][0. 0.]]
# [[0. 0.][0. 0.][0. 0.][0. 0.]]
# [[0. 0.][0. 0.][0. 0.][0. 0.]]]
np.zeros((3,))
=> This created 1-D array of 3 elements with zero valuenp.zeros((3, 4))
=> This created 2-D array of 3 rows and 4 columns with zero valuenp.zeros((3, 4, 2))
=> This created 3-D array of 3 rows, 4 columns, 2 layers with zero value
np.zeros()
with string data-type
Create NumPy Array using import numpy as np
arr_1d_zeros_str = np.zeros((3,), dtype=str) # 1-D array of str with 3 elements
print("arr_1d_zeros_str", arr_1d_zeros_str)
# Output
# arr_1d_zeros_str ['' '' '']
In this program, we have created NumPy array using np.zeros((3,), dtype=str)
with string data-type. As a result, an array with empty elements was created.
np.zeros()
with boolean data-type
Create NumPy Array using import numpy as np
arr_2d_zeros_bool = np.zeros((3, 4), dtype=bool) # 2-D array of bool with 3 rows and 4 columns
print("arr_2d_zeros_bool", arr_2d_zeros_bool)
# Output
# arr_2d_zeros_bool [[False False False False]
# [False False False False]
# [False False False False]]
In this program, we have created NumPy array using np.zeros((3, 4), dtype=bool)
with boolean data-type. As a result, an array with False
elements was created.
np.ones()
Create NumPy Array using np.ones()
creates NumPy array initialized all elements with one value. It is mainly used as placeholder value in matrix, and testing.
Syntax => ones(shape, dtype=None, order='C', *, like=None)
shape
(mandatory) – This is the shape or dimensions of an array to be created. An array can be created using 1-D, 2-D, 3-D or multi-dimensional array.dtype
(optional, default – float64) – The data type of an array can be int, float, or complex.order
(optional, default value – C) – The memory layout of the array can be C-style (row-major order) or F (Fortran-style (Column-major order).
import numpy as np
arr_1d_ones = np.ones((3,)) # 1-D array of ones with 3 elements
print("arr_1d_ones", arr_1d_ones)
arr_2d_ones = np.ones((3, 4)) # 2-D array of ones with 3 rows and 4 columns
print("arr_2d_ones", arr_2d_ones)
arr_3d_ones = np.ones((3, 4, 2)) # 3-D array of ones with 3 rows, 4 columns, 2 layers
print("arr_3d_ones", arr_3d_ones)
# Output
# arr_1d_ones [1. 1. 1.]
# arr_2d_ones [[1. 1. 1. 1.][1. 1. 1. 1.][1. 1. 1. 1.]]
# arr_3d_ones [[[1. 1.][1. 1.][1. 1.][1. 1.]]
# [[1. 1.][1. 1.][1. 1.][1. 1.]]
# [[1. 1.][1. 1.][1. 1.][1. 1.]]]
np.ones((3,))
=> This created 1-D array of 3 elements with one valuenp.ones((3, 4))
=> This created 2-D array of 3 rows and 4 columns with one valuenp.ones((3, 4, 2))
=> This created 3-D array of 3 rows, 4 columns, 2 layers with one value
np.ones()
with Boolean data-type
Create NumPy Array using import numpy as np
arr_2d_bool = np.ones((3, 4), dtype=bool) # 2-D array of 3 rows and 4 columns, with dtype=bool
print("arr_2d_bool", arr_2d_bool)
# Output
# arr_2d_bool [[ True True True True]
# [ True True True True]
# [ True True True True]]
In this program, we have created NumPy array using np.ones((3, 4), dtype=bool)
with boolean data-type. As a result, an array with True
elements was created.
np.ones()
with Complex data-type
Create NumPy Array using import numpy as np
arr_1d_complex = np.ones((3,), dtype=complex) # 1-D array of 3 elements, with dtype=complex
print("arr_1d_complex", arr_1d_complex)
# Output
# arr_1d_complex [1.+0.j 1.+0.j 1.+0.j]
In this program, we have created NumPy array using np.ones((3,), dtype=complex)
with complex data-type. As a result, an array with 1.+0.j
elements was created.
np.empty()
Create NumPy Array using np.empty()
is used to create an uninitialized array with random or garbage values. This function works faster than np.zeros()
and np.ones()
.
Syntax => empty(shape, dtype=None, order='C', *args, **kwargs)
import numpy as np
arr_1d_empty = np.empty((3,)) # 1-D empty array with 3 elements
print("arr_1d_empty", arr_1d_empty)
arr_2d_empty = np.empty((3, 4), dtype = int) # 2-D empty array with 3 rows and 4 columns
print("arr_2d_empty", arr_2d_empty)
# Output
# arr_1d_empty [1.13521682e-311 1.13521682e-311 0.00000000e+000]
# arr_2d_empty [[0 1076232192 -858993459 1077300428]
# [0 1077968896 0 1078231040]
# [0 1078591488 0 1078951936]]
This method output is not exactly defined. If we have previously created arrays then new array using np.empty()
can take previous array elements. In this program, we have 1-D and 2-D array using np.empty()
method.
np.full()
Create NumPy Array using np.full()
function is used to create NumPy array with specified value elements. All elements of this array will be a specific value. This array function initializes the array with constant values.
Syntax => numpy.full(shape, fill_value, dtype=None, order='C')
; where fill_value
is the value of elements in an array. All other parameters are same as defined previously.
import numpy as np
arr_1d_full = np.full((3,), 8) # 1-D array with 3 elements, fill value = 8
print("arr_1d_full", arr_1d_full)
arr_2d_full = np.full((3, 4), 6) # 2-D array with 3 rows and 4 columns, fill value = 6
print("arr_2d_full", arr_2d_full)
arr_3d_full = np.full((3, 4, 2), 5) # 3-D array with 3 rows, 4 columns, 2 layers, fill value = 5
print("arr_3d_full", arr_3d_full)
# Output
# arr_1d_full [8 8 8]
# arr_2d_full [[6 6 6 6][6 6 6 6][6 6 6 6]]
# arr_3d_full [[[5 5][5 5][5 5][5 5]]
# [[5 5][5 5][5 5][5 5]]
# [[5 5][5 5][5 5][5 5]]]
np.full((3,), 8)
=> This creates 1-D array with 3 elements and all elements of value 8.np.full((3, 4), 6)
=> This creates 2-D array with 3 rows and 4 columns and all elements of value 6.np.full((3, 4, 2), 5)
=> This creates 3-D array with 3 rows, 4 columns, 2 layers and all elements of value 5.
np.full()
with different data-type
Create NumPy Array using import numpy as np
arr_1d_full = np.full((3,), 8, dtype=float) # 1-D array with 3 elements, fill value = 8, data-type = float
print("arr_1d_full", arr_1d_full)
arr_2d_full = np.full((3, 4), 6.5, dtype=str) # 2-D array with 3 rows and 4 columns, fill value = 6.5, data-type = string
print("arr_2d_full", arr_2d_full)
# Output
# arr_1d_full [8. 8. 8.]
# arr_2d_full [['6' '6' '6' '6']
# ['6' '6' '6' '6']
# ['6' '6' '6' '6']]
np.full((3,), 8, dtype=float)
=> This creates 1-D array with 3 elements, all elements of value 8 and data-type float.np.full((3, 4), 6.5, dtype=str)
=> This creates 2-D array with 3 rows and 4 columns, all elements of value 6.5 and data-type string.
np.full()
with Complex value and float data-type
Create NumPy Array using import numpy as np
arr_1d_full_complex = np.full((3,), 8+6j, dtype=float) # 1-D, 3 elements, complex value, data-type = float
print("arr_1d_full_complex", arr_1d_full_complex)
# Output
# arr_1d_full_complex [8. 8. 8.]
# ComplexWarning: Casting complex values to real discards the imaginary part
# multiarray.copyto(a, fill_value, casting='unsafe')
In this program, we have created NumPy array using np.full((3,), 8+6j, dtype=float)
with complex value and float data-type. Complex values directly cannot be represented as float values. As a result, only the real part of complex numbers was taken and an array with 8.
elements was created. ComplexWarning
was raised – Casting complex values to real discards the imaginary part.
Conclusion
In conclusion, mastering the creation of NumPy arrays with predefined values is essential for efficient numerical computing in Python. Throughout this tutorial, we’ve explored various methods to initialize arrays, including using np.zeros()
to create arrays filled with zeros, np.ones()
for arrays filled with ones, and np.empty()
for uninitialized arrays that are faster to generate. We also covered how to use np.full()
to create arrays with a specified value, offering flexibility in array initialization. Understanding these functions enables you to create arrays tailored to your specific needs, optimizing performance and functionality in your data analysis and scientific computing tasks.
Function | Description |
---|---|
np.zeros() | Create an array filled with zeros. |
np.ones() | Create an array filled with ones. |
np.empty() | create an array with random or garbage values |
np.full() | Create an array filled with a specified value. |
Code snippets and programs related to Create Arrays with Predefined Values, can be accessed from GitHub Repository. This GitHub repository all contains programs related to other topics in NumPy tutorial.
Related Topics
- Introduction to NumPy: Core Features and Importance in different FieldsIntroduction to NumPy NumPy stands for Numerical Python. It is an open-source Python library, which is predominantly mostly used for numerical computations in Python. It provides mathematical functions applicable to work with multi-dimensional arrays and matrices. It is a powerful tool to handle large data structures and allows performing complex arithmetic calculations. It has built-in computational features…
- How to Install NumPy on Windows, Linux and Mac: step-by-step guideWe can install NumPy on all major operating systems such as Windows, Linux and macOS. NumPy installation process is very simple and can be done using pip, which is the Python package installer. Here are the steps to install NumPy on Windows, Linux and Mac operating systems. Install NumPy on Windows, Linux and Mac Prerequisites…
- NumPy Array in Python: First Step into Numerical PythonNumPy array in Python are basic entities for numerical computations. They originate from the NumPy library, an essential package in Python for scientific computing. A NumPy array also termed as ndarray, is highly efficient and an object of multi-dimensional array (N-dimensional array), which provides the base functions of numerical operations. Being NumPy Arrays an essential…
- numpy.array(): Create NumPy Arrays using Lists, Tuples and Arrays (with Example Programs)NumPy (Numerical Python) is one of the most powerful libraries for numerical computations, data manipulation, and analysis in Python. At the core of NumPy is the ndarray, a multi-dimensional or N-dimensional array that allows to store and manipulate large datasets efficiently. NumPy provides several ways to create arrays, from simple ones to more complex multi-dimensional…
- Create Arrays with Predefined Values using np.zeros(), np.ones(), np.full() and np.empty()Create Arrays with Predefined Values NumPy library provides various functions to create arrays with predefined values. While creating an array, these NumPy functions helps to initialize the arrays with initial values. In last tutorial, we learned about Key Features of NumPy Arrays in Python. In this article, we will learn about 4 functions to create…